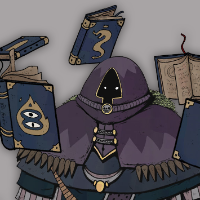
Hey! I've been making my own little character sheet for the past few weeks, and I just wanted to share a progress bar that I made. It's nothing revolutionary but it feels really smooth and provides a few features that a lot of people would want from a progress bar. I've been looking around the forums and people have asked for a progress bar like this, theorised about it, but never actually show a working example (as far as I know), so here it is:
HTML:
<input name="attr_hp_max" title="@{hp_max}" contenteditable="true" type="number" value="10">
<input name="attr_hp" title="@{hp}" contenteditable="true" type="number" value="10"> <div class="bar-track"> <input type="hidden" class="bar-value" name="attr_bar_value" value="20" /> <div class="bar-progress"></div> </div>
CSS:
/* ----- BAR TRACK ----- */ .charsheet .bar-track { background-color: #313031; /* Dark Grey */ width: 100px; height: 20px; border-radius: 5px; overflow: hidden; } /* ----- BAR PROGRESS ----- */ .charsheet .bar-progress { background-color: #77b05f; /* Green */ width: 100%; height: 20px; transition: width 0.25s ease-in-out, background-color 0.25s ease-in-out; } /* ----- BAR PROGRESS STEPS ----- */ .charsheet .bar-value[value^="-"] ~ div.bar-progress, .charsheet .bar-value[value="0"] ~ div.bar-progress { background-color: #da5b70; /* Red */ width: 0%; } .charsheet .bar-value[value^="0."] ~ div.bar-progress, .charsheet .bar-value[value="1"] ~ div.bar-progress, .charsheet .bar-value[value^="1."] ~ div.bar-progress { background-color: #da5b70; /* Red */ width: 5%; } .charsheet .bar-value[value="2"] ~ div.bar-progress, .charsheet .bar-value[value^="2."] ~ div.bar-progress { background-color: #da5b70; /* Red */ width: 10%; } .charsheet .bar-value[value="3"] ~ div.bar-progress, .charsheet .bar-value[value^="3."] ~ div.bar-progress { background-color: #da5b70; /* Red */ width: 15%; } .charsheet .bar-value[value="4"] ~ div.bar-progress, .charsheet .bar-value[value^="4."] ~ div.bar-progress { background-color: #da5b70; /* Red */ width: 20%; } .charsheet .bar-value[value="5"] ~ div.bar-progress, .charsheet .bar-value[value^="5."] ~ div.bar-progress { background-color: #da5b70; /* Red */ width: 25%; } .charsheet .bar-value[value="6"] ~ div.bar-progress, .charsheet .bar-value[value^="6."] ~ div.bar-progress { background-color: #e9a355; /* Yellow */ width: 30%; } .charsheet .bar-value[value="7"] ~ div.bar-progress, .charsheet .bar-value[value^="7."] ~ div.bar-progress { background-color: #e9a355; /* Yellow */ width: 35%; } .charsheet .bar-value[value="8"] ~ div.bar-progress, .charsheet .bar-value[value^="8."] ~ div.bar-progress { background-color: #e9a355; /* Yellow */ width: 40%; } .charsheet .bar-value[value="9"] ~ div.bar-progress, .charsheet .bar-value[value^="9."] ~ div.bar-progress { background-color: #e9a355; /* Yellow */ width: 45%; } .charsheet .bar-value[value="10"] ~ div.bar-progress, .charsheet .bar-value[value^="10."] ~ div.bar-progress { background-color: #e9a355; /* Yellow */ width: 50%; } .charsheet .bar-value[value="11"] ~ div.bar-progress, .charsheet .bar-value[value^="11."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 55%; } .charsheet .bar-value[value="12"] ~ div.bar-progress, .charsheet .bar-value[value^="12."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 60%; } .charsheet .bar-value[value="13"] ~ div.bar-progress, .charsheet .bar-value[value^="13."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 65%; } .charsheet .bar-value[value="14"] ~ div.bar-progress, .charsheet .bar-value[value^="14."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 70%; } .charsheet .bar-value[value="15"] ~ div.bar-progress, .charsheet .bar-value[value^="15."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 75%; } .charsheet .bar-value[value="16"] ~ div.bar-progress, .charsheet .bar-value[value^="16."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 80%; } .charsheet .bar-value[value="17"] ~ div.bar-progress, .charsheet .bar-value[value^="17."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 85%; } .charsheet .bar-value[value="18"] ~ div.bar-progress, .charsheet .bar-value[value^="18."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 90%; } .charsheet .bar-value[value="19"] ~ div.bar-progress, .charsheet .bar-value[value^="19."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 95%; } .charsheet .bar-value[value="20"] ~ div.bar-progress, .charsheet .bar-value[value^="20."] ~ div.bar-progress { background-color: #77b05f; /* Green */ width: 100%; }
SHEETWORKER:
<script type="text/worker"> on("change:hp change:hp_max sheet:opened", function() { update_progressBar(); });
var update_progressBar = function() {getAttrs(['hp', 'hp_max'], function(v) {const output = {};const hp_max = +v.hp_max || 0;const hp = +v.hp || 0;const hp_real = (hp > hp_max) ? hp_max : (hp < 0) ? 0 : hp;output.bar_value = (hp_real / hp_max) * 20;if (hp_real != hp) output.hp = hp_real;setAttrs(output);});};</script>
Of course big shoutout to Leothedino for the original CSS step concept. I took the idea and pushed it a bit further to fit what a lot of people seem to want from their custom progress bar. And thank you GiGs for the help with cleaning the code!