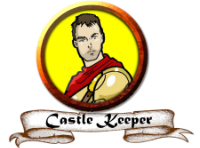
I am working on a modification of The Aaron's OnMyTurn script for a few reasons. 1. So characters can have multiple entries that automatically kick off without them all being entered into one ability. 2. So I can store different abilities into different categories so I can look at a character and go "oh this is a magical ability" or "oh this is a game function" etc for my own personal mental needs. 3. But mainly so I can have the character's action menu automatically whispered to them at the beginning of their turn. My question is fairly simple. Is there a way to amend the script so it pulls a macro/ability from a specific character sheet that everyone has access to? Or to write the Macro straight into the output of the API? Also, is there something in this API as written that sends to the chat twice and I am just not seeing it? on('ready', () => { const resolver = (token,character) => (text) => { const attrRegExp = /@{(?:([^|}]*)|(?:(selected)|(target)(?:\|([^|}]*))?)\|([^|}]*))(?:\|(max|current))?}/gm; const attrResolver = (full, name, selected, target, label, name2, type) => { let simpleToken = JSON.parse(JSON.stringify(token)); let charName = character.get('name'); type = ['current','max'].includes(type) ? type : 'current'; const getAttr = (n, t) => ( findObjs({type: 'attribute', name:n, characterid: character.id})[0] || {get:()=>getAttrByName(character.id,n,t)} ).get(t); const getFromChar = (n,t) => { if('name'===n){ return charName; } return getAttr(n,t); }; const getProp = (n, t) => { switch(n){ case 'token_name': return simpleToken.name; case 'character_name': return charName; case 'bar1': case 'bar2': case 'bar3': return simpleToken[`${n}_${'max'===t ? 'max' : 'value'}`]; } return getFromChar(n,t); }; if(name){ return getFromChar(name,type); } return getProp(name2,type); }; return text.replace(attrRegExp, attrResolver); }; const checkMenu = (obj,prev) => { let to=JSON.parse(obj.get('turnorder')||'[]'); let toPrev=JSON.parse(prev.turnorder||'[]'); if(to.length && to[0].id!=='-1' && to[0].id !== (toPrev[0]||{}).id){ let token = getObj('graphic',to[0].id); if(token && token.get('represents')){ let character = getObj('character',token.get('represents')); let ability = findObjs({ name: 'Menu', characterid: character.id }, {caseinsensitive: true})[0]; if(ability){ let content = resolver(token,character)(ability.get('action')).replace(/\[\[\s+/g,'[['); try { sendChat(character.get('name'),content); } catch(e){ log(`Menu: ERROR PARSING: ${content}`); log(`Menu: ERROR: ${e}`); } } } } }; on( 'change:campaign:turnorder', (obj,prev)=>setTimeout(()=>checkMenu(Campaign(),prev),1000) ); on('chat:message', (msg) => { if('api'===msg.type && /^!eot\b/.test(msg.content)){ setTimeout(()=>checkMenu(Campaign(),{turnorder:JSON.stringify([{id:-1}])}),1000); } }); }); The specific character/ability I am looking to pull from is a character named Macros and the ability is called Menu .