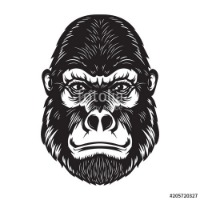
Hi! I'm currently working on an importer for npc data. When it's ready the players (or the GM) can simply copy a creature from a sourcebook (PDF) and copy it into a textfeld in the character sheet that then translates the data into a creature and fills the npc character sheet with the given data. This worked fine for attributes but with skills I'm getting a strange error. The click listener for the import button delivers the content of the npc.skills array that I filled beforehand with the skills. This looks like this: As you can see every array element is one skill separated with a whitespace from it's value. The method importNsc then splits every array element at the position of the whitespaces and separates skill and value: function importNsc(nsc) { let vAttributes = ["ausstrahlungnsc","beweglichkeitnsc","intuitionnsc","konstitutionnsc","mystiknsc","staerkensc","verstandnsc","willenskraftnsc","groessenklassensc","geschwindigkeitnsc","lebenspunktensc","fokusnscgesamt","verteidigungnsc","schadensreduktionnsc","koerperlicherwiderstandnsc","geistigerwiderstandnsc"]; let vSkills = {"anführen":"anfuehren","geschichte und mythen":"geschichteundmythen","länderkunde":"laenderkunde","schlösser und fallen":"schloesserundfallen","straßenkunde":"strassenkunde","zähigkeit":"zaehigkeit","stärkungsmagie":"staerkungsmagie"}; let newRowId; let update = {}; let temp = ""; for (var i = 0; i < nsc.attributes.length; i++) { let attribute = vAttributes[i]; let value = nsc.attributes[i]; update[attribute] = +value; } nsc.fertigkeiten[0] = " ".concat(nsc.fertigkeiten[0]); for (var i = 0; i < nsc.fertigkeiten.length; i++) { let skill = nsc.fertigkeiten[i].split(" ")[1]; let value = parseInt(nsc.fertigkeiten[i].split(" ")[2], 10) || 0; if (skill in vSkills) { skill = vSkills[skill]; } console.log("skill:" + skill + ",value:" + value); newRowId = generateRowID(); update['repeating_fertigkeitennsc_' + newRowId + '_fertigkeitnsc'] = skill; update['repeating_fertigkeitennsc_' + newRowId + '_fertigkeitswertnsc'] = +value; } setAttrs(update); } The repeating_row (repeating_fertigkeitennsc) looks like this: It just consists of a select element for the skill, a text field for the value, a roll button and a checkbox for a special skill mode. In code: <fieldset class="repeating_fertigkeitennsc"> <select name="attr_fertigkeitnsc""> <option value="akrobatik">Akrobatik</option> <option value="alchemie">Alchemie</option> <option value="anfuehren">Anf&uuml;hren</option> <option value="arkanekunde">Arkane Kunde</option> <option value="athletik">Athletik</option> <option value="darbietung">Darbietung</option> <option value="diplomatie">Diplomatie</option> <option value="edelhandwerk">Edelhandwerk</option> <option value="empathie">Empathie</option> <option value="entschlossenheit">Entschlossenheit</option> <option value="fingerfertigkeit">Fingerfertigkeit</option> <option value="geschichteundmythen">Geschichte &amp; Mythen</option> <option value="handwerk">Handwerk</option> <option value="heilkunde">Heilkunde</option> <option value="heimlichkeit">Heimlichkeit</option> <option value="jagdkunst">Jagdkunst</option> <option value="laenderkunde">L&auml;nderkunde</option> <option value="naturkunde">Naturkunde</option> <option value="redegewandtheit">Redegewandtheit</option> <option value="schloesserundfallen">Schl&ouml;sser &amp; Fallen</option> <option value="schwimmen">Schwimmen</option> <option value="seefahrt">Seefahrt</option> <option value="strassenkunde">Stra&szlig;enkunde</option> <option value="tierfuehrung">Tierf&uuml;hrung</option> <option value="ueberleben">&Uuml;berleben</option> <option value="wahrnehmung">Wahrnehmung</option> <option value="zaehigkeit">Z&auml;higkeit</option> <option value="nix">------ Magieschulen ------</option> <option value="bannmagie">Bannmagie</option> <option value="beherrschungsmagie">Beherrschungsmagie</option> <option value="bewegungsmagie">Bewegungsmagie</option> <option value="erkenntnismagie">Erkenntnismagie</option> <option value="felsmagie">Felsmagie</option> <option value="feuermagie">Feuermagie</option> <option value="heilungsmagie">Heilungsmagie</option> <option value="illusionsmagie">Illusionsmagie</option> <option value="kampfmagie">Kampfmagie</option> <option value="lichtmagie">Lichtmagie</option> <option value="naturmagie">Naturmagie</option> <option value="schattenmagie">Schattenmagie</option> <option value="schicksalsmagie">Schicksalsmagie</option> <option value="schutzmagie">Schutzmagie</option> <option value="staerkungsmagie">St&auml;rkungsmagie</option> <option value="todesmagie">Todesmagie</option> <option value="verwandlungsmagie">Verwandlungsmagie</option> <option value="wassermagie">Wassermagie</option> <option value="windmagie">Windmagie</option> </select> <input type=number name="attr_fertigkeitswertnsc" value="0" min="0"> <button type="action" name="act_skill" class="sheet-ability-button">0</button> <input type="checkbox" name="attr_gm" value="1" /> </fieldset> But I noticed that Roll20 gives me an error everytime I click on the Import button (only the first time though, when I click it a second time, everything works fine): This is my debug output followed by the error. The debug output looks fine, there doesn't seem to be anything wrong. Now comes the strange part: The said error occurs exactly three times during the import and always on the exact same three skills (akrobatik, entschlossenheit, zaehigkeit). If I remove those three skills from the import string everything works smoothly. Additionally I also add this three skills to the same repeating section on sheet:opened and there they don't produce any errors: getSectionIDs("fertigkeitennsc", function(idarray) { if (idarray.length == 0) { var newrowattrs = {}; var newrowid = generateRowID(); newrowattrs["repeating_fertigkeitennsc_" + newrowid + "_fertigkeitnsc"] = "akrobatik"; <------ newrowid = generateRowID(); newrowattrs["repeating_fertigkeitennsc_" + newrowid + "_fertigkeitnsc"] = "athletik"; newrowid = generateRowID(); newrowattrs["repeating_fertigkeitennsc_" + newrowid + "_fertigkeitnsc"] = "entschlossenheit"; <----- newrowid = generateRowID(); newrowattrs["repeating_fertigkeitennsc_" + newrowid + "_fertigkeitnsc"] = "heimlichkeit"; newrowid = generateRowID(); newrowattrs["repeating_fertigkeitennsc_" + newrowid + "_fertigkeitnsc"] = "wahrnehmung"; newrowid = generateRowID(); newrowattrs["repeating_fertigkeitennsc_" + newrowid + "_fertigkeitnsc"] = "zaehigkeit"; <----- setAttrs(newrowattrs); } }); (FYI: This is because this are the default skills that every npc has, so I add them anyway if the repeating row is empty because the gm has to add them anyway - but removing this code doesn't make any difference). I'm coming to the following conclusion: Since the error only occurs the first time I import the skills and it doesn't occur again on further imports unless I reload the page it must have something to do with uninitialized objects. But I really don't know what's wrong with this three specific skills that cause this error. I double checked the entries in the <input> object but they're exactly the same. I know this is a terribly specific problem but maybe somebody has a hint for me. Cheers!