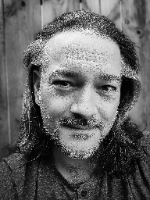
Hello script wizards! I am trying to write my first script! Huzzah! But it is not working...booo! I am basing it on the framework for @Eric D's Automatic Temp HP Management (from THIS POST from over 10 years ago) that watches for changes in the weightttotal attribute value to check for Encumbrance. Eric D's TempHP script looks for changes in the HP bar and checks for the existance of temp_hp, then offsets the HP changes accordingly. Likewise if it sees a value in the hp_temp attribute, it assigns a status marker to reflect it. It is a slick script that my party uses often (Paladin and Way of the Long Death Monk). What I want it to do is check for changes in the weightttotal attribute and check for existance of encumbrance attribute. If the Encumbrance attribute value is 1, it should apply a status marker. If it is 0, it should remove the status marker. <<EDIT -- HERE IS MY PROBLEM>> The encumbrance attribute is NaN, so it is not detecting it based on the attribute value. I have tried substituting the Encumbrance attribute for a simple Strength attribute and multipying that times 15 to get the encumbrance threshold, but it seems my coding skills are lacking to pull that off. Since I do not want it to do anything to HP or any bar value, I have removed all references to such in my version of the script, but have otherwise left the framework the same, subbing out the attribute names and syntax to apply to the encumbrance and weight attributes. To my mind it should be a simple change to Eric D's script, but I cannot get it to parse the change in weighttotal attribute value. What am I doing wrong? Code: /* global TokenMod, ChatSetAttr */
on('ready', () => {
// Configuration parameters
const EncMarker = 'SpeedDown::3155973';
const Weight = 'weighttotal';
const EncAttributeName = 'encumbrance';
/////////////////////////////////////////////
const clearURL = /images\/4277467\/iQYjFOsYC5JsuOPUCI9RGA\/.*.png/;
const unpackSM = (stats) => stats.split(/,/).reduce((m,v) => {
let p = v.split(/@/);
let n = parseInt(p[1] || '0', 10);
if(p[0].length) {
m[p[0]] = Math.max(n, m[p[0]] || 0);
}
return m;
},{});
const packSM = (o) => Object.keys(o)
.map(k => ('dead' === k || true === o[k] || o[k]<1 || o[k]>9) ? k : `${k}@${parseInt(o[k])}` ).join(',');
const checkEnc = (obj) => {
let v = parseFloat(obj.get('current'));
findObjs({
type: 'graphic',
represents: obj.get('characterid')
})
.filter( (t) => t.get(lnk) !== '')
.filter( (t) => !clearURL.test(t.get('imgsrc') ) )
.forEach((g)=>{
let sm = unpackSM(g.get('statusmarkers'));
if(v>0){
sm[EncMarker]=v;
} else {
delete sm[EncMarker];
}
g.set({
statusmarkers: packSM(sm)
});
});
};
const assureEncMarkers = () => {
let queue = findObjs({
type: 'attribute',
name: EncAttributeName
});
const burndownQueue = ()=>{
if(queue.length){
let attr = queue.shift();
checkEnc(attr);
setTimeout(burndownQueue,0);
}
};
burndownQueue();
};
const temporalEncCache = {};
const accountForWeightChange = (obj,prev) => {
// 1. did weighttotal change and is it a scale
const weighttotal = parseInt(obj.get(value),10);
let weight = parseInt(obj.get(value),10);
const diff = weight-parseFloat(prev[attr]);
if( !isNaN(weighttotal) && diff !== 0 ) {
let changes = {};
// 2. does it represent a character
// 3. does the hp bar represent an attribute
const character = getObj('character',obj.get('represents'));
if( diff < 0 && character && obj.get(lnk)!=='' ){
// 4. is there encumbrance
const encumbrance = findObjs({
type: 'attribute',
characterid: character.id,
name: EncAttributeName
})[0];
if( encumbrance ) {
const now = Date.now();
}
}
let sm = unpackSM(obj.get('statusmarkers'));
changes.statusmarkers = packSM(sm);
obj.set(changes);
}
};
const onAttributeChange = (obj) => {
if(obj.get('name') === EncAttributeName){
checkEnc(obj);
}
};
on("change:attribute", onAttributeChange);
on("change:token", accountForWeightChange);
if('undefined' !== typeof TokenMod && TokenMod.ObserveTokenChange){
TokenMod.ObserveTokenChange(accountForWeightChange);
}
if('undefined' !== typeof ChatSetAttr && ChatSetAttr.registerObserver){
ChatSetAttr.registerObserver('change',onAttributeChange);
}
assureEncMarkers();
});