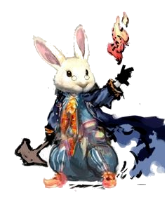
So I know I need to edit this line (90): var pickone = randomInteger(tips.length) - 1; But I know nothing about json. Trying to repurpose this and need it to go in order. Code: /*
Roll 20 - Script
Title: DnD 5e Tips Based Loading Page
Author: Michael Nekrasov
Description:
Creates a page to drop players on to that gives helpful tips while DM sets up a map
To use:
1. Create a page called "Loading" (you can change this in variables)
2. On the object layer create a textbox (will be auto created if none exists)
Note:
To optimize resources, the tooltips only change when the players (not GM) are viewing the page
*/
var displaySpeed = 15000; //Sets speed (in milleseconds) at which to cycle tips
var numChars = 70; //Set maximum number of characters per line
var targetPageName = "Loading"; //The name of the page you want to use for your loading screen
var onlyChangeIfVisible = false; //Only rotates tips when players are on Loading screen
var debug = true; //Enables debugging messages
var tips = [
"In the quietude of their separate lives, five souls moved through mundane rhythms until an inexplicable event shattered their reality. A blinding light enveloped them, revealing forgotten memories and distant echoes. When their vision returned, they stood in a dimly lit chamber, strangers bound by shared bewilderment.",
"Who were they? Why were they here? The questions echoed silently, etching themselves into their newfound reality. As their eyes adjusted to the gloom, they realized they were not alone.",
"Five figures stood there, drawn together by an invisible thread. And so began their tale—the tale of five souls awakened, their destinies irrevocably intertwined, and the promise of a world beyond imagination awaiting them",
];
//
// Don't edit bellow this line if you don't know what you are doing
// ------------------------------------------------------
function fixNewObject(obj)
{
var p = obj.changed._fbpath;
var new_p = p.replace(/([^\/]*\/){4}/, "/");
obj.fbpath = new_p;
return obj;
}
on("ready", function(obj) {
var loadingPage;
// Warn user that there is no page
if( findObjs({_type: "page",name: targetPageName}).length == 0){
log("Warning! No Page named '"+targetPageName+"' exists, create one for this script to work");
return;
}
else{
loadingPage = findObjs({_type: "page",name: targetPageName})[0];
}
//Update the loading screen with a new tip (requires players to be viewing page)
var UpdateWithNewTip = function() {
//Change Tip only if Red player marker is on Loading Page
if(onlyChangeIfVisible && getObj("page", Campaign().get("playerpageid")).get("name") != targetPageName) return;
else if(debug) log("Players not on "+targetPageName);
var textObjects = findObjs({
_type: "text",
_pageid: loadingPage.get("_id"),
layer: "objects"
});
if(debug) log("Text Objects on page");
if(debug) log(textObjects);
//Find First text field or create one if none exists
var text;
if(textObjects.length == 0){
if(debug) log("Create New Text Box");
text = createObj("text", {
_pageid: loadingPage.get("_id"),
left: 650,
top: 255,
width: 200,
height: 200,
font_size: 56,
text: "Loading...",
layer: "objects"
});
text = fixNewObject(text);
}
else{
if(debug) log("Text Box Already Exists");
text = textObjects[0];
}
var pickone = randomInteger(tips.length) - 1;
var formattedText;
var formattedLines = [];
_.each(tips[pickone].split(/\n/),function(l){
formattedText = '';
_.each(l.split(''),function(c){
if(formattedText.length > numChars && ' ' === c) {
formattedLines.push(formattedText);
formattedText = '';
} else {
formattedText += c;
}
});
formattedLines.push(formattedText);
});
formattedText=formattedLines.join("\n");
text.set("text", formattedText);
if(debug) log( "new tip: "+ text.get("text"));
}
UpdateWithNewTip();
setInterval( UpdateWithNewTip, displaySpeed); //take an action every ___ seconds
});