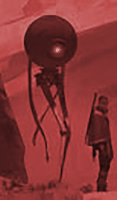
They don't have token IDs. So how can we select one programmatically? By color maybe? That would be convenient. Use case: I want to move a light barrier as I move a token on the objects layer. This will allow enemy ships (or our own) to hide behind things like asteroids. Further in depth... I have a radar scope, and I wanted to simulate ship movement. To accomplish this, I move all token that are on the GM or objects layer that include certain bar 2 values in the opposite direction from the one the Ship pilot indicates (see below). Those too far away (at the edge of the scope) are moved to the gmlayer to not mess with the Battle UI appearance. I manually move them back to the objects layer if they are discovered. All good, right? It works beautifully, but not with an object that blocks light. For that, I need to move those walls/one-ways just like any other token on my list. "But how?", he asks... tokens.forEach(token => {
const bar3 = token.get('bar3_value');
if (['obstacle', 'ship', 'base', 'torpedo', 'moon', 'planet','los'].includes(bar3) && token.get('name') !== 'Ship') {
// Move the token according to the direction
const currentX = token.get('left');
const currentY = token.get('top');
const offsetX = offsets[direction].x;
const offsetY = offsets[direction].y;
token.set({
left: currentX + offsetX,
top: currentY + offsetY
});
// Check distance from the "Ship" token
const distance = Math.sqrt(Math.pow((token.get('left') - ship.get('left')), 2) + Math.pow((token.get('top') - ship.get('top')), 2));
if (distance > 12.5 * hexSize) {
token.set('layer', 'gmlayer', 'walls');
}
}