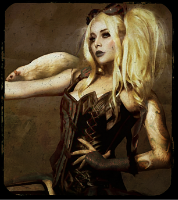
Hello all ! I'm currently making a small custom sheet for my campaign and I got to the point where I would need some basic Javascript. I thought it would not be too much trouble to implement but I guess making it works in Roll20 is not as easy as planned unfortunately ... Basically I have several cells with skills levels in them and I would like their background color to change depending on the level of the related input value in the cell. So on a normal web page this would be the code I use : HTML : <div class="sheet-cell">Fencing<input type="number" name="attr_Fencing" value="0" min="0" max="3"></div> <div class="sheet-cell">Archery<input type="number" name="attr_Archery" value="0" min="0" max="3"></div> <div class="sheet-cell">Witchcraft<input type="number" name="attr_Witchcraft" value="0" min="0" max="3"></div> <!-- Other skills .... --> CSS : .sheet-cell.bg-color-0 { background-color: white;}
.sheet-cell.bg-color-1 { background-color: lightyellow;}
.sheet-cell.bg-color-2 { background-color: lightgreen;}
.sheet-cell.bg-color-3 { background-color: green;} Script : <script> function updateCellBackground(input) { const cell = input.closest('.sheet-cell'); const value = parseInt(input.value); cell.classList.remove('bg-color-0', 'bg-color-1', 'bg-color-2', 'bg-color-3'); cell.classList.add(`bg-color-${value}`); } const inputs = document.querySelectorAll('.cell input[type="number"]'); inputs.forEach(input => { updateCellBackground(input); input.addEventListener('input', () => updateCellBackground(input)); }); </script> Now, I've tried to adapt the script for Roll20 and this is what I came up with at the moment : <script type="text/worker">
function updateCellBackground(attributeName) {
getAttrs([attributeName], function(values) {
const value = parseInt(values[attributeName]) || 0;
const cellClass = `bg-color-${value}`;
const cellElement = document.querySelector(`[name="attr_${attributeName}"]`).closest('.sheet-cell');
cellElement.classList.remove('bg-color-0', 'bg-color-1', 'bg-color-2', 'bg-color-3');
cellElement.classList.add(cellClass);
});
}
// Skills to check
const attributes = ['Fencing', 'Archery', 'Witchcraft']; // Other skills ...
attributes.forEach(attr => {
on(`change:${attr}`, function() {
updateCellBackground(attr);
});
});
on('sheet:opened', function() {
attributes.forEach(attr => {
updateCellBackground(attr);
});
});
</script> And I didn't have much success since ... To be totally honest I'm not even sure if that's supposed to work and I'm kind of stuck ... Can this script work or am I making mistakes somewhere ? Is there another solution, maybe an easier one, to get the wanted result in this case ? If you have any advice or insight they would be very welcomed ! Thanks in advance all :) !