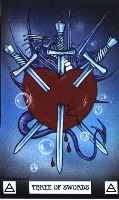
Greetings!
After manually opening doors and moving Dynamic Lighting objects for a few game sessions, I decided I needed to bump to Mentor to automate it. Unfortunately I wasn't completely happy with Matt's implementation that can be found in the link below. (I didn't like the whole "gear thing" and I don't need the traps feature).
So I had to write my own.
This is my first time using JavaScript so I know there is probably a better way to do it. But my programming experience is limited to some VBA coding for Excel and when I used to write in COBOL and FORTRAN77 many years ago. So while not a complete noob, my knowledge of modern language structures, coding concepts, naming standards, etc is minimal.
Thus I warn you the code is VERY ugly. I'm not even sure I should share it. I just figured it it helps one other person, it's worth posting.
If the excellent coders on site could want to clean it up, make it more robust, add some error checking, etc, that's fine.
So w/o further ado...
Script Code
/*
SimpleDoorControls Use
Creating a linked door system
-----------------------------
Verify you're on the Token/Object Layer.
Place a graphic of a door or other barrier in the closed position.
Name that token DoorClosed (all one word, and yes the D and C need to be capitalized).
Place a graphic of a door in the desired open position.
Name that token DoorOpen (all one word, and yes the D and O need to be capitalized).
Draw a line to act as the Dynamic Lighting barrier for the door when closed (while still on the Token layer).
Select all three objects.
Type !DoorsLink (or set it as a macro and use that macro)
The Open Door and Dynamic Lighting barrier will be moved to the GM layer and Dynamic Lighting layer, respectively. The open and closed doors will be renamed. This is how they are linked.
DO NOT RENAME THE LINKED OBJECTS.
Do this for each set of doors needed.
Opening and Closing doors
-------------------------
Once the objects are linked
Select the door on the token layer
Type !DoorOpenClose
The door will open if it is closed, or it will close if it is opened.
*/
on("chat:message", function (msg) {
var DoorOpenID;
var DoorClosedID;
var PathID;
var cmdNames = ["!DoorsLink","!DoorOpenClose"];
switch(msg.content.split(" ")[0]) {
case "!DoorsLink":
//make sure three items are selected
try {
if (msg.selected.length != 3) throw "invalid selection";
} catch (err) {
sendChat("Doors", "/w GM Select and place a graphic named 'DoorClosed', a graphic named 'DoorOpen' and a path to use as a wall.");
break;
}
//identify and get the ID for each each type of selected item
_.each(msg.selected, function(obj) {
try {
var o = getObj(obj["_type"], obj["_id"]);
if (o.get("_type") == "graphic" && o.get("name") == "DoorOpen") {
DoorOpenID = o.get("_id");
} else if (o.get("_type") == "graphic" && o.get("name") == "DoorClosed") {
DoorClosedID = o.get("_id");
} else if (o.get("_type") == "path") {
PathID = o.get("_id");
}
} catch (err) { }
});
//move each item to the appropriate layer &&
//rename each door with the ID of the Path followed by the ID of the other door
_.each(msg.selected, function(obj) {
try {
var o = getObj(obj["_type"], obj["_id"]);
if (o.get("_type") == "graphic" && o.get("name") == "DoorOpen") {
o.set("name",PathID + " " + DoorClosedID);
o.set("layer","gmlayer");
} else if (o.get("_type") == "graphic" && o.get("name") == "DoorClosed") {
o.set("name",PathID + " " + DoorOpenID);
} else if (o.get("_type") == "path") {
o.set("layer","walls");
}
} catch (err) { }
});
break;
case "!DoorOpenClose":
_.each(msg.selected, function(obj) {
try {
var o = getObj(obj["_type"], obj["_id"]);
var currDoorID = o.get("_id");
o.set("layer","gmlayer");
//Determine ID of other two objects
var str = o.get("name");
var pathID = str.slice(0,20);
var otherDoorID = str.slice(21,41);
//Move other objects to appropriate layers
var otherDoor = getObj("graphic",otherDoorID);
otherDoor.set("layer","objects");
var Path = getObj("path",pathID);
var currPathLayer = Path.get("layer");
if (currPathLayer == "walls") {
Path.set("layer","gmlayer");
} else {
Path.set("layer","walls");
}
} catch (err) { }
});
break;
}
});