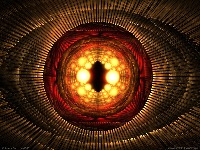
I got the following code from another thread and made some minor modifications: on("chat:message", function(msg) { // looks for !~ and converts it to Earthdawn Step dice. if(msg.type == "api" && msg.content.indexOf("!step") !== -1) { var stepNum = eval(msg.content.replace("!step", " ")); if (stepNum < 2){ sendChat(msg.who, "Step 1"); sendChat(msg.who, "/r 1d4!-2"); } else if (stepNum === 2){ sendChat(msg.who, "Step 2"); sendChat(msg.who, "/r 1d4!-1"); } else if (stepNum === 3){ sendChat(msg.who, "Step 3"); sendChat(msg.who, "/r 1d4!"); } ... else { sendChat(msg.who, "Step Number " + stepNum + " is not valid"); } } }); Essentially in Earthdawn you roll a set of exploding dice depending on a step number. The code goes to step 40 but I just cut out the middle. It works great for standard rolls. I'll probably clean up the code a bit more but it is functional. From here I can throw it into macros if I want. Easy enough. But I want two more versions of this basic idea and I really don't know where to start. First I want a version where the result is shown only to me (the GM). I don't need to see the full die roll laid out, just the resulting number. More specifically I want players to initiate the roll but have the result only visible to the GM. I think /gmroll displays to the player and GM so that isn't quite what I want. I'm guessing that I need to store the result in a variable instead of using sendChat and then "/w gm "+result but perhaps that is the dumb way to do things and it isn't clear to me how to store the result of an exploding die roll in a variable without going to the chat window. Second I want to roll the step die and use that for initiative. Adding &{tracker} to the end of the roll didn't work. I've looked at more complex initiative scripts but they seem to be doing a lot of stuff so I'm not sure where the initiative piece is happening. It seems like I need to again store the result in a variable and pass that variable into a function. But which function and how to store that variable I don't know. I don't know javascript at all but I am familiar with scripting in general but I am having a hard time figuring out what functions form the API I should be employing to do this stuff. Any help would be greatly appreciated!