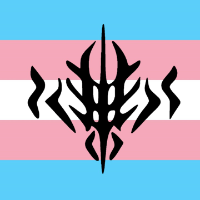
This is my first script in the API, so it's not much. I am very open to constructive criticism from all of you! And if someone other than me actually finds this useful and would like additional features then please post and I will see what I can do.
Purpose: This script is intended to manage the base Diplomacy DC and attitudes of NPC characters in the Pathfinder system. It assumes the Pathfinder character sheets are being used and will use the current CHA-mod for a character. It will create any attributes that are not present assuming a default attitude of "Indifferent" and a CHA score of 12. If you wish a character to start with an attitude other than "Indifferent" you will need to manually create a "Disposition" attribute on the character and set it to the desired value.
Commands:
!diplomacyCheck
This command will whisper the GM the current disposition and base Diplomacy DC of an NPC represented by a selected token.
!diplomacyCheck score
If you include a numeric score representing the Diplomacy Check score of a PC trying to change the attitude of the NPC the script will instead compare that score to the DC of the NPC represented by the token you have selected and change that NPC's attitude accordingly. The script will whisper the results of the check to the GM.
Code: Download from GitHub
Purpose: This script is intended to manage the base Diplomacy DC and attitudes of NPC characters in the Pathfinder system. It assumes the Pathfinder character sheets are being used and will use the current CHA-mod for a character. It will create any attributes that are not present assuming a default attitude of "Indifferent" and a CHA score of 12. If you wish a character to start with an attitude other than "Indifferent" you will need to manually create a "Disposition" attribute on the character and set it to the desired value.
Commands:
!diplomacyCheck
This command will whisper the GM the current disposition and base Diplomacy DC of an NPC represented by a selected token.
!diplomacyCheck score
If you include a numeric score representing the Diplomacy Check score of a PC trying to change the attitude of the NPC the script will instead compare that score to the DC of the NPC represented by the token you have selected and change that NPC's attitude accordingly. The script will whisper the results of the check to the GM.
Code: Download from GitHub