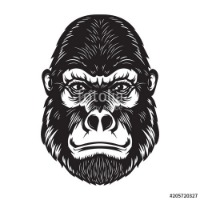
Hi! I have the following structure to get all the names of all rows of a repeating section (with abilities that buff the character): on("change:repeating_superpowers", function(eventinfo) {
var superpowers = new Array();
superpowers.push("test1");
getSectionIDs("repeating_superpowers", function(idarray) {
_.each(idarray, function(currentID, i) {
getAttrs(["repeating_superpowers_" + currentID +"_spvalue", "repeating_superpowers_" + currentID +"_spname"], function(v) {
var superpowername = v["repeating_superpowers_" + currentID + "_spname"];
var superpowervalue = v["repeating_superpowers_" + currentID + "_spvalue"];
superpowers.push("test2");
if (superpowername.toLowerCase() == "lasereyes") {
setAttrs({
lasereyes: 1
});
} else if (superpowername.toLowerCase() == "wings") {
setAttrs({
speed: 10
});
}
});
});
});
setAttrs({
testfield: superpowers.length
});
}); Let's assume that the attribute names and the fields are correct (because it works basically). What I want is to get an array that holds the names of all (e.g.) super powers. I think it should work the way it is right now, but if I try the code, it writes "1" in "testfield", but it should write 2. So I guess that there is a problem with the scope of the array superpowers. But since the variable is declared right in the beginning, I don't have a clue why it shouldn't work. Can anyone give me a helpful tip regarding this problem? Greetings, Loki