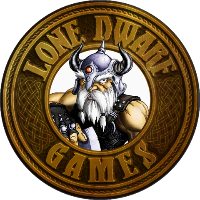
v2.0 Added support to toggle the token between object and gmlayer. Allows the light to have a physical form such as lightning. Made this for my Pathfinder Iron God's game. Someone might find it useful. Leigh //==============================================================================================
//
// FlickeringLights.js (v2.0)
//
// Simple script that takes all the graphic tokens named 'FlickeringLight' on the currently
// active PLAYERS PAGE and causes them to blink on/off. The blinking is done by toggling the
// 'All Players See Light' on the token (Edit Token->Advanced->Emits Light).
//
// The token can also be toggled between the object and and gmlayer if 'Aura 1 Square' is
// checked ('Edit Token->Basic->Aura 1 Square'). I used this to make my lightning image flash
// with the ligh.
//
// How often the light blinks can be configured using 'Bar 1' (Edit Token->Basic->Bar 1). The
// value is considered a percentage where 90 would mean that the light will be on 90% of the
// time. The default is 50 if no value is found in 'Bar 1'.
//
// The rate of how often all the FlickeringLights are updated can be changed using the speed
// command followed milliseconds. So if you set the speed to say 5000, then all the lights will
// have a CHANCE to be toggled every 5 seconds. I use it with a very fast update like 100ms and
// have most of the lights use a high percentage in 'Bar 1' like 90. This makes the all the
// lights stay on most of the time and blink off for a very short period of time to simulate
// something like a crashed spaceship lighting.
//
// Chat Commands:
//
// !FlickeringLights help
// !FlickeringLights on
// !FlickeringLights off
// !FlickeringLights speed [ms]
// !FlickeringLights enable [group]
// !FlickeringLights disable [group]
//
//
// Based on the Jack T FlicheringLights script posted on the API forum.
// (<a href="https://app.roll20.net/forum/post/1366850/flickering-lights" rel="nofollow">https://app.roll20.net/forum/post/1366850/flickering-lights</a>)
//
// Used help_menu from Steve Kootz's DynamicLightHelper
// (<a href="https://github.com/Roll20/roll20-api-scripts/tree/master/DynamicLightHelper" rel="nofollow">https://github.com/Roll20/roll20-api-scripts/tree/master/DynamicLightHelper</a>)
//==============================================================================================
var FlickeringLights =
{
SCRIPT_NAME : "FlickeringLight.js",
CHAT_COMMAND : "!FlickeringLights ",
TOKEN_NAME : "FLight",
IntervalMS : 200,
Timer : null,
ChatTemplate : _.template( "/w GM <div style=\"padding:1px 3px; border: 1px solid <%=border%>; background: <%=background%>; color: <%=color%>; font-size: 80%;\"><%=text%><br><br></div>" ),
//==============================================================================================
//==============================================================================================
onUpdate : function()
{
var tokens = findObjs(
{
_pageid: Campaign().get( "playerpageid" ),
_type: "graphic",
_subtype: "token",
} );
for( var index = 0; index < tokens.length; ++index )
{
var token = tokens[index];
var name = token.get( "name" );
// Is it a FlickeringLight?
if ( name.indexOf( FlickeringLights.TOKEN_NAME ) != 0 )
continue;
// Is disabled?
if ( token.get( "aura2_square" ) == true )
continue;
// Percent chance on.
var chanceOn = token.get( "bar1_value" );
if ( chanceOn.length == 0 )
chanceOn = 50;
var bOn = ((Math.random() * 100.0) < chanceOn) ? true : false;
token.set( "light_otherplayers", bOn );
if ( token.get( "aura1_square" ) == true )
{
if ( bOn == true )
{
token.set( "layer", "objects" );
}
else
{
token.set( "layer", "gmlayer" );
}
}
}
},
//==============================================================================================
//==============================================================================================
onDisableAll : function( groupName, bDisable )
{
var tokens = findObjs(
{
_pageid: Campaign().get( "playerpageid" ),
_type: "graphic",
_subtype: "token",
} );
for( var index = 0; index < tokens.length; ++index )
{
var token = tokens[index];
var name = token.get( "name" );
var idxTokenName = name.indexOf( FlickeringLights.TOKEN_NAME );
if ( idxTokenName == -1 )
continue;
var idxGroupName = name.indexOf( groupName, idxTokenName + FlickeringLights.TOKEN_NAME.length );
var tokenGroupName = name.slice( idxGroupName );
if ( tokenGroupName == groupName )
{
log( "Disable [" + tokenGroupName + "]: " + bDisable );
token.set( "light_otherplayers", (bDisable == false) ? true : false );
token.set( "aura2_square", bDisable );
}
}
},
//==============================================================================================
//==============================================================================================
start : function()
{
clearInterval( FlickeringLights.Timer );
FlickeringLights.Timer = setInterval( function() { FlickeringLights.onUpdate(); }, FlickeringLights.IntervalMS );
log( "StartFlickering()" );
},
//==============================================================================================
//==============================================================================================
stop : function()
{
if ( FlickeringLights.Timer )
{
clearInterval( FlickeringLights.Timer );
FlickeringLights.Timer = null;
log( "StopFlickering" );
}
},
//==============================================================================================
//==============================================================================================
showHelp : function ()
{
sendChat( FlickeringLights.SCRIPT_NAME,
FlickeringLights.ChatTemplate(
{
border: "#00529B",
background: "#BDE5F8",
color: "#00529B",
text: "<b>Help:</b><br>" +
"<dl>" +
"<dt>on</dt><dd>Start updating lights</dd>" +
"<dt>off</dt><dd>Stop updating lighting</dd>" +
"<dt>speed [ms]</dt><dd>Change the rate of update in milliseconds</dd>" +
"<dt>enable [group]</dt><dd>Start flickering tokens named '" + FlickeringLights.TOKEN_NAME + "' the group name.</dd>" +
"<dt>disable [group]</dt><dd>Stop flickering tokens named '" + FlickeringLights.TOKEN_NAME + "' the group name.</dd>" +
"</dl> "
} ));
},
//==============================================================================================
//==============================================================================================
onApiCommand : function( command )
{
log( "OnApiCommand: " + command );
if ( !command.indexOf( "help" ) )
{
FlickeringLights.showHelp();
}
else
if ( !command.indexOf( "on" ) )
{
FlickeringLights.start();
}
else
if ( !command.indexOf( "off" ) )
{
FlickeringLights.stop();
}
else
if ( !command.indexOf( "speed" ) )
{
var speed = command.slice( 6 )
FlickeringLights.IntervalMS = Number( speed );
FlickeringLights.start();
}
else
if ( !command.indexOf( "enable" ) )
{
var groupName = command.slice( 7 )
FlickeringLights.onDisableAll( groupName, false );
}
else
if ( !command.indexOf( "disable" ) )
{
var groupName = command.slice( 8 )
FlickeringLights.onDisableAll( groupName, true );
}
},
};
//==================================================================================================
//==================================================================================================
on( "chat:message", function( msg )
{
try
{
switch( msg.type )
{
case "api":
var idxCmd = msg.content.indexOf( FlickeringLights.CHAT_COMMAND );
if ( idxCmd != -1 )
FlickeringLights.onApiCommand( msg.content.slice( idxCmd + FlickeringLights.CHAT_COMMAND.length ) );
break;
}
}
catch( err )
{
sendChat( "*** FlickeringLights Exception: ", err.message );
}
} );
log( "FlickeringLights COMPILED [" + (new Date()) + "]");