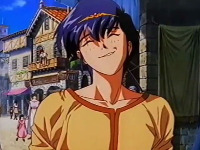
I am attempting to set the value of an attribute, but it doesn't seem to be working. At the same time, though, the log doesn't return any errors and everything else seems to be working fine up to that point. Here is my relevant code: //credit to Brian on the forums for the basic function framework!
on('chat:message', function(msg) {
if (msg.type != 'api') return;
var parts = msg.content.split(' ');
var command = parts.shift().substring(1);
if (command == 'script') {
if (parts.length < 2) {
sendChat('SYSTEM', 'You must provide a selected token id and a target token id.');
return;
}
var selectedId = parts[0];
var targetId = parts[1];
var selectedToken = getObj('graphic', selectedId);
var targetToken = getObj('graphic', targetId);
if (!selectedToken) {
sendChat('SYSTEM', 'Selected token id not provided.');
return;
}
if (!targetToken) {
sendChat('SYSTEM', 'Target token id not provided.');
return;
}
var who = getObj('character', selectedToken.get('represents'));
if (!who) {
who = selectedToken.get('name');
} else {
who = 'character|' + who.id;
}
var attacker = getObj('character', selectedToken.get('represents'));
var defender = getObj('character', targetToken.get('represents'));
sendChat(who, '/me attacks ' + targetToken.get('name') + '!');
//Grab basic stats- this isn't relevant here
//Hit/crit/avo/dod
let HitA = getAttrByName(attacker.id, 'hit');
let HitB = getAttrByName(defender.id, 'hit');
let CritA = getAttrByName(attacker.id, 'crit');
let CritB = getAttrByName(defender.id, 'crit');
let AvoA = getAttrByName(attacker.id, 'avo');
let AvoB = getAttrByName(defender.id, 'avo');
let DdgA = getAttrByName(attacker.id, 'lck_total');
let DdgB = getAttrByName(defender.id, 'lck_total');
let DmgtypeA;
let DmgtypeB;
let DmgA;
let DmgB;
//Targeted stat
//The numeric values of DmgA and DmgB are calculated here
//Actual battle script- This is the part that doesn't work
//Check if attacker's attack hits
if (randomInteger(100) < (HitA - AvoB)){
sendChat(who,"/me 's attack hits!")
//Check if attack crits
if (randomInteger(100) < (CritA - DdgB)){
DmgA *= 3;
sendChat(who,"Crit!")
}
HPB -= DmgA;
log(HPB);
//this doesn't work for some reason
defender.set("HP_current", HPB)
} else {
sendChat(who,"/me misses!")
}
}
});