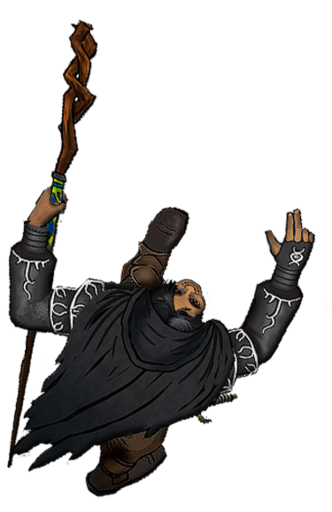
Version: 1.1 I have made a few changes to the Bloodied and Dead Status Markers script from <a href="https://wiki.roll20.net/API:Cookboox" rel="nofollow">https://wiki.roll20.net/API:Cookboox</a> . You do have now the following settings: var lifeBarNumber = 3; // 3:red; 2:blue; 1:green
var percentForRedmark = 50; // Write for example 50 for 50%
var totalKillThreshold = -10; // As soon as a tokens life goes bellow this value it gets an additional (killed) symbol.
That means, that you can select which bare you
would like to use as your life bar. It allows you to select the %-level after
which a token is seen as wounded. It gives you the ability to select an ending
level. Which means, after this amount of negative hit points a target
counts as completely dead (no reanimation).
Additional to that, you get a symbol if a
token has been reanimated (his life points have been below 1 and now are
again higher than 0). This symbol disappears once the token reaches again
max heal.
If you do find any bugs let me know.
on("change:graphic", function(obj) {
// Settings:
var lifeBarNumber = 3; // 3:red; 2:blue; 1:green
var percentForRedmark = 50; // Write for example 50 for 50%
var totalKillThreshold = -10; // As soon as a tokens life goes bellow this value
// it gets an additional (killed) symbol.
// Is life bar set at all?
// If not simply escape the function.
if(obj.get("bar" + lifeBarNumber + "_max") === "") return;
// Back from the dead?
if((obj.get("status_dead") == true || obj.status_skull == true) && obj.get("bar" + lifeBarNumber + "_value") > 0){
SetStatuSymbolById(obj,3,1);
}
// Total-kill
if(obj.get("bar" + lifeBarNumber + "_value") <= totalKillThreshold){
SetStatuSymbolById(obj,0,0);
SetStatuSymbolById(obj,1,1);
SetStatuSymbolById(obj,2,1);
SetStatuSymbolById(obj,3,0);
}
// Dead
else if(obj.get("bar" + lifeBarNumber + "_value") <= 0){
SetStatuSymbolById(obj,0,0);
SetStatuSymbolById(obj,1,1);
SetStatuSymbolById(obj,2,0);
SetStatuSymbolById(obj,3,0);
}
// Bellow x% life
else if(obj.get("bar" + lifeBarNumber + "_value") <= obj.get("bar" + lifeBarNumber + "_max") / 100 * percentForRedmark) {
SetStatuSymbolById(obj,0,1);
SetStatuSymbolById(obj,1,0);
SetStatuSymbolById(obj,2,0);
}
else{
ResetDeathSymbols(obj);
// Back from the dead but now again with full (or beyond that) health?
// TODO: >= not working..?
if(obj.get("bar" + lifeBarNumber + "_value") == obj.get("bar" + lifeBarNumber + "_max")){
SetStatuSymbolById(obj,3,0);
}
}
});
/*
Reset the following symboles
redmarker, dead & skull
*/
function ResetDeathSymbols(obj){
SetStatuSymbolById(obj,0,0);
SetStatuSymbolById(obj,1,0);
SetStatuSymbolById(obj,2,0);
}
/*
Sets the value of a given tokens symbol by the symbols id
symbolid:
0:redmarker
1:dead
2:skull
3:death-zone
x:y
value:
true/1 or false/0
*/
function SetStatuSymbolById(obj, symbolid, value) {
switch(symbolid){
case 0:
SetStatusSymbolByName(obj, "redmarker", value);
break;
case 1:
SetStatusSymbolByName(obj, "dead", value);
break;
case 2:
SetStatusSymbolByName(obj, "skull", value);
break;
case 3:
SetStatusSymbolByName(obj, "death-zone", value);
break;
default:
// Something is wrong so log it and exit.
log("Error: SetStatuSymbolById - There was no usable number for symbolid given!");
log(" Object name: " + obj.get("name") + "; symbolid: " + symbolid + "; value: " + value);
return;
}
}
/*
Sets the value of a given tokens symbol by the symbols name
value:
true/1 or false/0
*/
function SetStatusSymbolByName(obj, symbolname, value){
value = CheckForTrueOrFalse(value);
// Set the symbols value.
obj.set("status_" + symbolname, value);
}
/*
Checks if a given value is true or false.
value:
0:false
1:true
default:false
*/
function CheckForTrueOrFalse(value){
if (value === 0){value = false;}
else if (value === 1){value = true;}
else if (value === true || value === false){
// Nothing to do, these are valid values.
}
// The input for value is not known, therefore we set it to false.
else{
value = false;
log("Error: CheckForTrueOrFalse - An unhandled value has been used!");
log(" value has been set to de default value " + value);
}
return value;
}
/*
Debug function
Place where ever you would like to see the actual values.
*/
function DebugMe(obj,msg){
log("--- DEBUG: " + msg + "---");
log("obj name: " + obj.get("name"));
log("status values:");
log("redmarker: " + obj.get("status_redmarker"));
log("dead: " + obj.get("status_dead"));
log("skull: " + obj.get("status_skull"));
log("death: " + obj.get("status_death"));
} By the way: does any one of you know why this is not working or how I could get it to work? // Sets the value of a given tokens symbol by the symbols name
// value: true/1 or false/0
//
// Actuall not in use, due to parsing problems...
function SetSymbolByName(obj, symbolname, value){
if (value == 0){value = false;}
else if (value == 1){value = true;}
else if (value == true || value = false){
// Nothing to do, these are valid values.
}
else{value = true;}
// Set the symbols value.
//obj.set({ symbolname: value });
obj[symbolname] = value;
//log(obj.get("name") + " " + symbolname + " " + value);
}