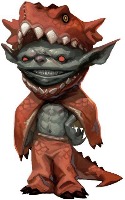
I've been working on a script for my players to spend/add money to their cash. Ive got most of it worked out other than internal 'make change' part of it. Example 1: Player spends 3 gold and they have only 1 gold piece, but have 5 platinum: Make it take the other 2 gold off the plat and set them to 4 plat, 8 gold. Example 2: Player spends 3 gold but have 0 plat, or gold, but have 50 silver. Make it take 30 silver, and set them to 20 silver left. (above examples are all using the 1:10 ratio of pathfinder for PP:GP:SP:CP) I thought of having it do the math to figure out a decimal total value of PP for both owned and spent money..but then it 'magically' exchanges coins to the cleanest sum amount in return..IE (an extreme example): They spend 5 gold, they have 0 PP, 2 GP, 6080SP, 30 CP. It was figuring out they wanted to spend 0.5 PP, and their wallet was worth 7.03 PP. SO it would take the money (leaving them with 6.53 PP) and give them 6PP, 5 GP, 3SP back (not good at all) I cannot think of a way to do this, without a metric ton of if/else if/else loops. There has to be an easier way I'm not grasping here. This is what I have so far..the "do magic" inside the " TakeMoney " fuction part is where I am stuck. Anyone have any tips? on ( 'chat:message' , function ( msg ) { /*["!coins","Spend","0PP","0GP","0SP","0CP"]*/ "use strict" ; if (msg.type === "api" && msg.content. indexOf ( "!coins" ) !== - 1 ) { var cWho = findObjs ({ type: 'character' , name: msg.who })[ 0 ]; if (cWho === undefined ) { cWho = RollRight (msg.playerid); //outside function---- } msg.who = cWho. get ( "name" ); var oPP = findObjs ({ name: "PP" , type: "attribute" , characterid: cWho.id }, { caseInsensitive: true })[ 0 ]; var oGP = findObjs ({ name: "GP" , type: "attribute" , characterid: cWho.id }, { caseInsensitive: true })[ 0 ]; var oSP = findObjs ({ name: "SP" , type: "attribute" , characterid: cWho.id }, { caseInsensitive: true })[ 0 ]; var oCP = findObjs ({ name: "CP" , type: "attribute" , characterid: cWho.id }, { caseInsensitive: true })[ 0 ]; if (oPP === undefined || oGP === undefined || oSP === undefined || oCP === undefined ) { sendChat ( 'Coin Purse' , "Not All Coins Set" ); return ; } var msgFormula = msg.content. split ( / \s + / ); var Money = msgFormula. slice ( Math . max (msgFormula.length - 4 , 1 )); var spent = "" ; Money. forEach ( function ( type ) { //type sent as array format ["0PP","0GP","0SP","0CP"] var coinType = type. replace ( / [ ^ a-zA-Z] + / g , '' ); var coinCount = type. replace ( / [ ^ 0-9] / g , '' ); if (coinCount !== '0' ) { if (msgFormula[ 1 ] == "Spend" ) { TakeMoney (cWho, coinType, coinCount); } else { AddMoney (cWho, coinType, coinCount); } var addtext = coinCount + "" + coinType + " " ; spent = spent + addtext; } }); var currentPP = parseInt (oPP. get ( "current" ), 10 ); var currentGP = parseInt (oGP. get ( "current" ), 10 ); var currentSP = parseInt (oSP. get ( "current" ), 10 ); var currentCP = parseInt (oCP. get ( "current" ), 10 ); var coinSum = "Wallet: " + currentPP + "PP, " + currentGP + "GP, " + currentSP + "SP, " + currentCP + "CP" ; var chatmsg = "&{template:pf_block}{{color=darkgrey}}{{name=" + msgFormula[ 1 ] + ":" + spent + "}}{{description=" + coinSum + "}}" ; sendChat ( 'Coin Purse' , "/w " + msg.who + " " + chatmsg); if (msg.who !== "GM" ) { sendChat ( 'Coin Purse' , "/w GM " + chatmsg); } } }); /*---SPEND MONEY---*/ function TakeMoney ( cWho , type , amount ) { var oC = findObjs ({ name: type, _type: "attribute" , characterid: cWho.id }, { caseInsensitive: true })[ 0 ]; var currentCoins = parseInt (oC. get ( "current" ), 10 ); var spentCoins = parseInt (amount, 10 ); if (currentCoins < spentCoins) { spentCoins = spentCoins - currentCoins; //(do magic with remaining amount to spend)... } else { var total = parseInt (currentCoins - spentCoins, 10 ); oC. setWithWorker ( 'current' , total); } } /*---ADD MONEY---*/ function AddMoney ( cWho , type , amount ) { var oC = findObjs ({ name: type, _type: "attribute" , characterid: cWho.id }, { caseInsensitive: true })[ 0 ]; var currentCoins = parseInt (oC. get ( "current" ), 10 ); var newCoins = parseInt (amount, 10 ); var total = parseInt (currentCoins + newCoins, 10 ); oC. setWithWorker ( 'current' , total); }