Here it is. Use the API command:
!claim-character SOME TEXT
It will find any characters that have the supplied text (case-insensitive) contiguously, ignoring punctuation or spaces, for example:
!claim-character bob the
would match any of:
- "Bob the Slayer"
- "Mr Bob the happy"
- "thisbobthekiller"
It will then whisper a list of the matching characters with a claim button:
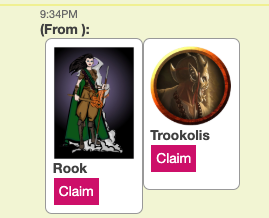
If there are no matches, it will tell you:
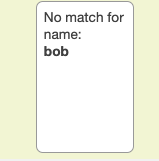
Clicking a "Claim" button will assign the character to the person clicking it (and put it in their journal), and whisper a link to the player and notice they claimed the character, as well as whisper the GM to let them know the player claimed it:
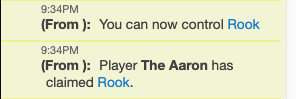
Probably having a macro with:
!claim-character ?{character name}
for your players would make this pretty easy.
Code:
on('ready',()=>{
const keyname = t => t.replace(/[^a-z0-9]/ig,'').toLowerCase();
const s = {
choice: "max-width: 6em; border: 1px solid #999; border-radius: .5em; background-color: white; padding: .5em;float:left;min-height: 10em;",
nomatch: "max-width: 6em; border: 1px solid #999; border-radius: .5em; background-color: white; padding: .5em;float:left;min-height: 10em;",
image: 'display: inline-block;',
name: 'display: block; font-weight: bold;',
message: '',
button: ''
};
const choice = c=>`<div style="${s.choice}"><img style="${s.image}" src="${c.get('avatar')}" /><div style="${s.name}">${c.get('name')}</div><div style="${s.button}"><a href="!claim-character-by-id ${c.id}">Claim</a></div></div>`;
const nomatch = n=>`<div style="${s.nomatch}"><div style="${s.message}">No match for name:</div><div style="${s.name}">${n}</div></div>`;
on('chat:message', msg => {
if('api'===msg.type) {
if(/^!claim-character\b\s/i.test(msg.content)){
let who = getObj('player',msg.playerid).get('_displayname');
let output='';
let name = msg.content
.split(/\s+/)
.slice(1)
.join(' ')
;
let key = keyname(name);
findObjs({
type: 'character'
})
.filter(c=>-1 !== (keyname(c.get('name'))).indexOf(key))
.forEach(c=> output += choice(c));
if(!output.length){
output += nomatch(name);
}
sendChat('',`/w "${who}" <div style="${s.container}">${output}<div style="clear:both;"></div></div>`);
}
if(/^!claim-character-by-id\b\s/i.test(msg.content)){
let who = getObj('player',msg.playerid).get('_displayname');
let args = msg.content.split(/\s+/);
let character = getObj('character', args[1]);
if(character){
character.set({
controlledby: `${[...new Set([...character.get('controlledby').split(','), msg.playerid])].join(',')}`,
inplayerjournals: `${[...new Set([...character.get('inplayerjournals').split(','), msg.playerid])].join(',')}`
});
sendChat('',`/w "${who}" You can now control <a style="color: #07c;" href="http://journal.roll20.net/character/${character.id}">${character.get('name')}</a>`);
sendChat('',`/w gm Player <b>${who}</b> has claimed <a style="color: #07c;" href="http://journal.roll20.net/character/${character.id}">${character.get('name')}</a>.`);
} else {
sendChat('',`/w "${who}" No character found for <code>${args[1]}</code>.`);
}
}
}
});
});