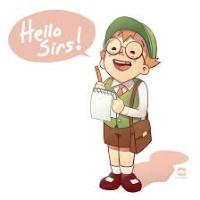
Forgive me, I'm easily stumped on scripting stuff. I've been working on a script that handles only one dice roll and passes that to split arguments. I'm now trying to add a damage roll to the same script and I'm not sure how you differentiate it. Currently my script has: let hits = 0; let dice = []; let cf = 0; // walk the inline roll structure grabbing the dice and comparing vs target, focus and expertise (msg.inlinerolls || []).forEach(r => { ((r.results || {}).rolls || []).forEach(r2 => { (r2.results || []).forEach(r3 => { hits += ((r3.v || 0) <= target ? 1 : 0); hits += ((r3.v || 0) <= focus ? 1 : 0); cf += ((r3.v||0)>= (expertise === 0 ? 19: 20) ? 1 : 0); dice.push(r3.v || 0); }); }); And that's the only use of "dice." So let's say my chat input is: !infattack ?{Dice|2}d20 @{selected|weapon_damage_rep}d6 How do I grab the second roll and do separate things with it? Sorry, I know I should be able to find an easy answer but so far I have beefed it...