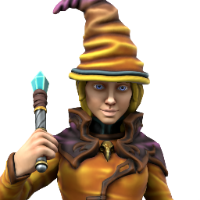
Hi,
Similar to what Simone did in this thread, I've created some javascript code to help sort through lengthy macro bars. First, the disclaimer: you need to know how to "install" javascript user-scripts to your browser. (Try googling "javascript userscript browser" with your browser name included. FYI, Chrome handles it natively and Firefox needs an extension. Not sure about the others.) And you'll most likely be wanting all of your players to do the same... so chances are good that unless the macro bars are really bugging you, or you already know how to do this, then this isn't worth it for you. Nevertheless.
So assuming that's all good, this can do one or both of two things.
1. If you add certain key text to your macro names, it can add labels and force line breaks.
2. If you fill in images that you want for certain button names, it will add them when it finds a match.
So for example if your Abilities looks like this:
<(Attributes)>Agility
Smarts
Spirit
Strength
Vigour)>
<(Skills)>Notice
Channelling
Throwing
Knife/Sling-Damage
Untrained
(The labels must have this "<(label)>" at the start and the line break must be at the end like this ")>").
and you make sure to fill in the icons section similarly to this:
var icons = [];
icons["Agility"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
icons["Smarts"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
icons["Spirit"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
.
.
.
icons["Untrained"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
then the script will do this:
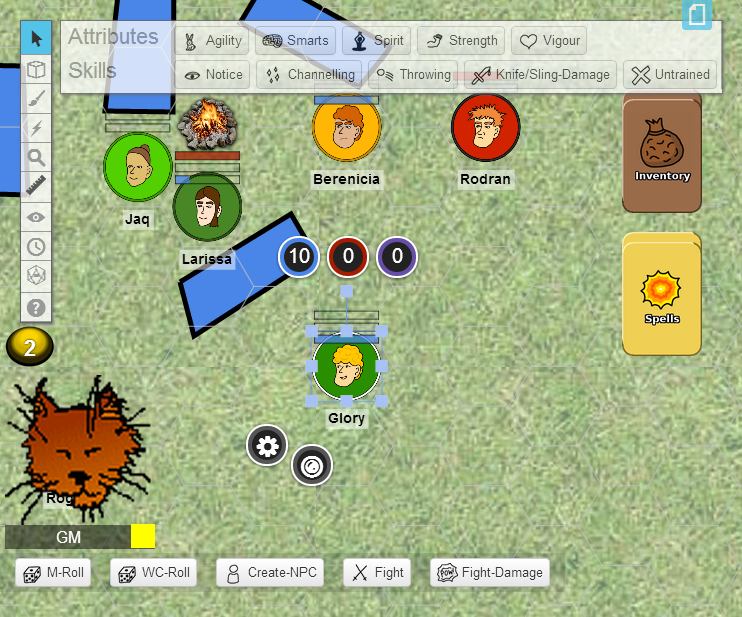
PS. Line breaks aren't handled in the lower area macros, but labels still show up OK.
Here's the script:
and here are some icons I drew that you could use to get started, if you like.
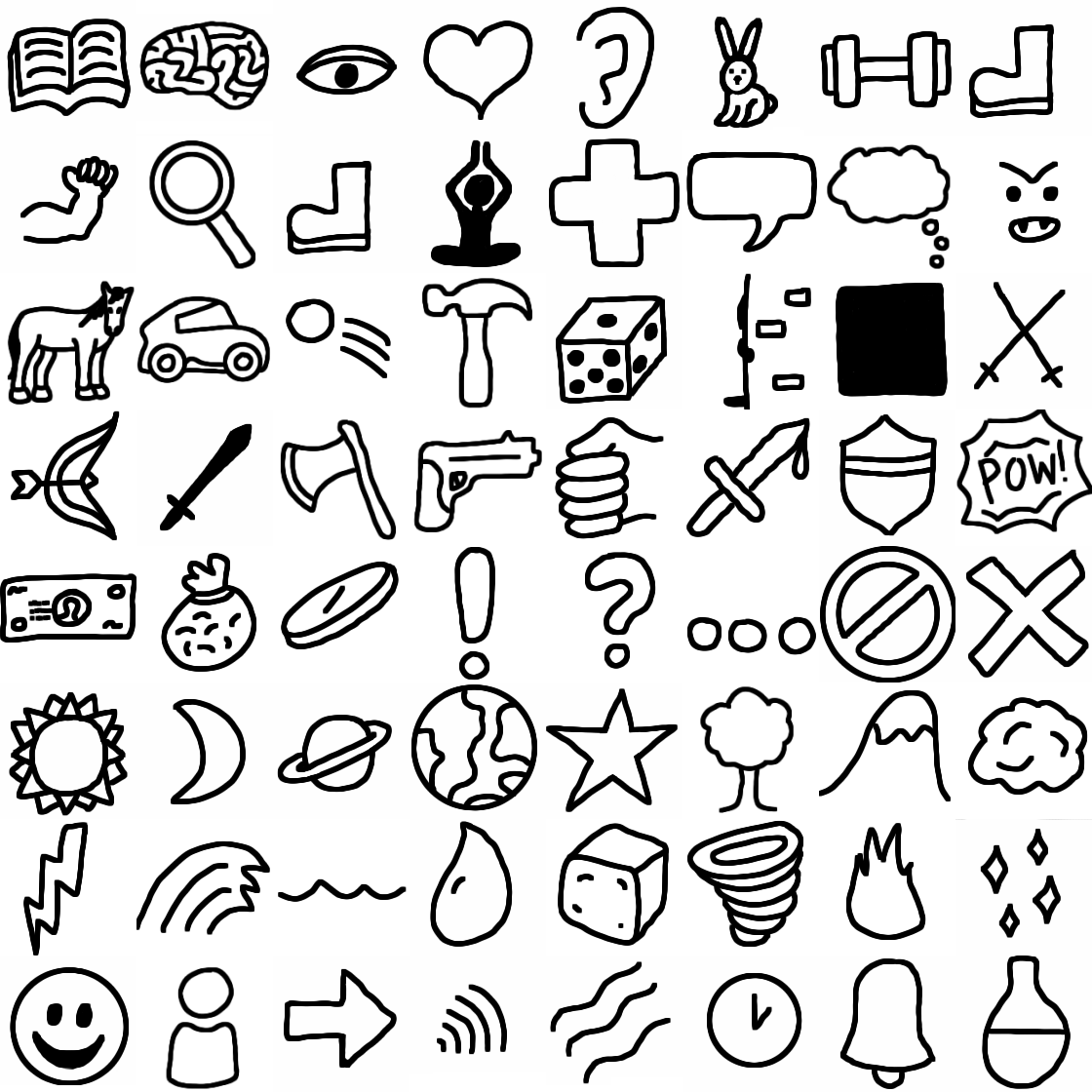
Similar to what Simone did in this thread, I've created some javascript code to help sort through lengthy macro bars. First, the disclaimer: you need to know how to "install" javascript user-scripts to your browser. (Try googling "javascript userscript browser" with your browser name included. FYI, Chrome handles it natively and Firefox needs an extension. Not sure about the others.) And you'll most likely be wanting all of your players to do the same... so chances are good that unless the macro bars are really bugging you, or you already know how to do this, then this isn't worth it for you. Nevertheless.
So assuming that's all good, this can do one or both of two things.
1. If you add certain key text to your macro names, it can add labels and force line breaks.
2. If you fill in images that you want for certain button names, it will add them when it finds a match.
So for example if your Abilities looks like this:
<(Attributes)>Agility
Smarts
Spirit
Strength
Vigour)>
<(Skills)>Notice
Channelling
Throwing
Knife/Sling-Damage
Untrained
(The labels must have this "<(label)>" at the start and the line break must be at the end like this ")>").
and you make sure to fill in the icons section similarly to this:
var icons = [];
icons["Agility"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
icons["Smarts"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
icons["Spirit"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
.
.
.
icons["Untrained"] = "https://s3.amazonaws.com/files.d20.io/images/1234/ABCDE/thumb.png?123";
then the script will do this:
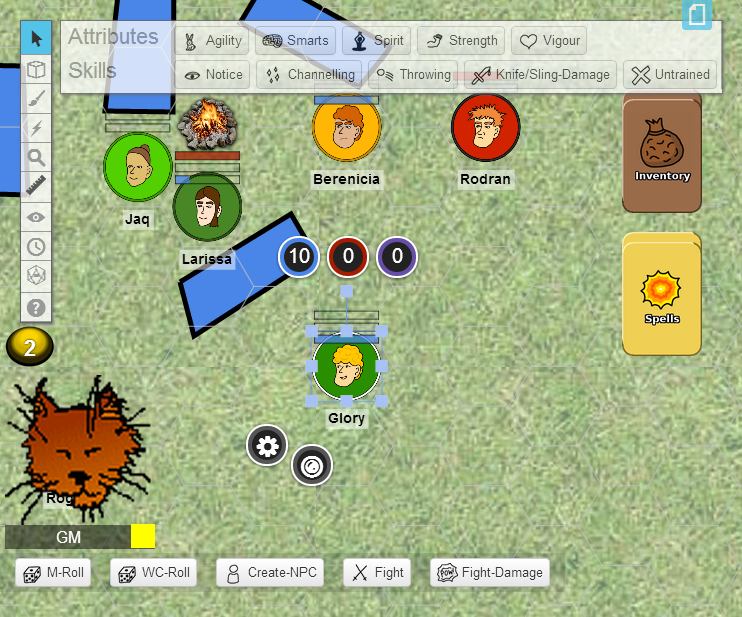
PS. Line breaks aren't handled in the lower area macros, but labels still show up OK.
Here's the script:
// ==UserScript==
// @name Roll20 Macro Prettifier
// @namespace parmeisan
// @version 0.1
// @description Adds images and labels to the macro lines.
// @require http://ajax.googleapis.com/ajax/libs/jquery/1/jqu...
// @match https://app.roll20.net/editor/
// @copyright 2013, parmeisan
// ==/UserScript==
// Icon definition array ************ Fill in your image list here ************
var icons = [];
icons["Agility"] = "https://s3.amazonaws.com/files.d20.io/images/<Fill-in-your-info-here>";
setInterval(prettifyMacros, 500);
function isnull(o) { return typeof o == 'undefined' || o == null || o.length == 0; };
function prettifyMacros()
{
var all_buttons = $("button[data-type=ability]").add("button[data-type=macro]").add(".macrobox > button");
// Looks for keywords in ability names and replaces with newlines and labels.
// - For a newline, add ")>" at the END of an ability name, e.g. "Strength)>"
// - For a label, add "<(label)>" at the START of an ability name, e.g. "<(Skills:)>Perception"
all_buttons.each(function ()
{
var text = $(this).html();
if (text.indexOf(")>") == text.length - 5)
{
text = text.replace(")>", "");
$(this).html(text);
$(this).after('<ul style="display: block;">');
}
if (text.indexOf("<(") == 0)
{
var pos = text.indexOf(")>");
var b_text = text.substring(5, pos);
var h_text = text.substring(pos+5);
$(this).before("<div class=macro-label style='display: inline-block; padding: 0px 15px 0px 5px;'>" + b_text + "</div>");
$(this).html(h_text);
}
});
// Line them up better
var max_w = 20;
$(".macro-label").each(function ()
{
var this_w = $(this).width();
if (this_w > max_w) max_w = this_w;
});
$(".macro-label").css("min-width", max_w + "px");
// Add Icons
all_buttons.each(function ()
{
var style = "width:21px; height:21px; padding: 0px; vertical-align: middle;"
var btn = $(this);
var img = icons[btn.html()];
btn.css("line-height", "1em");
btn.css("height", "29px");
btn.css("padding", "4px 6px");
btn.css("display", "inline-block");
if (!isnull(img)) btn.html("<img style='" + style + "' src='" + img + "' />" + " " + btn.html());
});
}
and here are some icons I drew that you could use to get started, if you like.
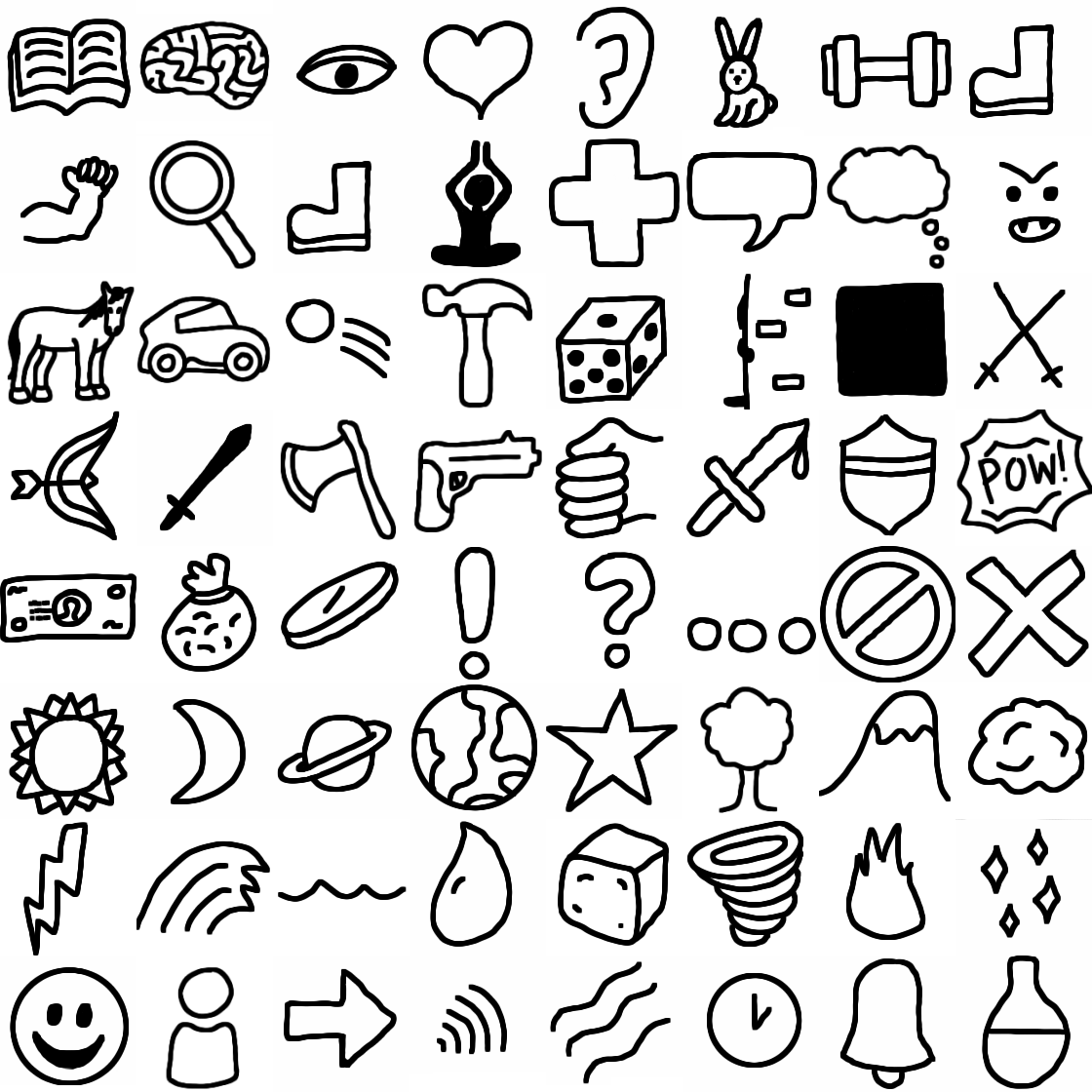