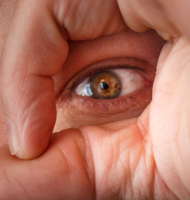
I've got a bit of code that handles some simple attribute calcs triggered using change:repeating_weapons, and this seems to work just fine as long I make a change to an attribute within repeating_weapons. As it should.
But, I would also like to run this function on sheet:opened as well as change:strength_total (and a few other non-repeating attributes, but I'll keep it simple for now.)
If I add sheet:opened and change:strength_total, I get a log error
SHEET WORKER ERROR: You attempted to set an attribute beginning with 'repeating_' but did not include a Row ID or Attribute Name in repeating_weaponseating_weapons_weapon_skill_tot
SHEET WORKER ERROR: You attempted to set an attribute beginning with 'repeating_' but did not include a Row ID or Attribute Name in repeating_weaponseating_weapons_weapon_bonus_tot
SHEET WORKER ERROR: You attempted to set an attribute beginning with 'repeating_' but did not include a Row ID or Attribute Name in repeating_weaponseating_weapons_weapon_damage_tot
I'm guessing this means I need to add some additional js to grab and insert the rowID for the setAttrs? Is that necessary, if so, can you post an example? If it's not necessary, how can run these calcs on repeating_weapons if any of my desired events are met?
TIA for any help you can offer.
code that works for changes within repeating_weapons
/* set weapon bonus and damage totals */ on("change:repeating_weapons", function () { console.log(">>>> Change Detected: Weapon Attacks - recalculating totals <<<<"); getAttrs(['repeating_weapons_weapon_skill', 'repeating_weapons_weapon_skill_tot', 'repeating_weapons_weapon_bonus', 'repeating_weapons_weapon_bonus_tot', 'repeating_weapons_weapon_misc_attack','repeating_weapons_weapon_bonus_max', 'repeating_weapons_weapon_damage', 'repeating_weapons_weapon_misc_damage', 'repeating_weapons_weapon_damage_tot', 'strength_total', 'agility_total', 'marksmanship', 'melee'], function (values) { const weapon_skill = parseInt(values.repeating_weapons_weapon_skill, 10) || 0, weapon_bonus = parseInt(values.repeating_weapons_weapon_bonus, 10) || 0, weapon_misc_attack = parseInt(values.repeating_weapons_weapon_misc_attack, 10) || 0, weapon_bonus_max = parseInt(values.repeating_weapons_weapon_bonus_max, 10) || 0, weapon_damage = parseInt(values.repeating_weapons_weapon_damage, 10) || 0, weapon_misc_damage = parseInt(values.repeating_weapons_weapon_misc_damage, 10) || 0, attribute = (weapon_skill === 0 ? parseInt(values.strength_total, 10) || 0 : parseInt(values.agility_total, 10) || 0), skill = (weapon_skill === 0 ? parseInt(values.melee, 10) || 0 : parseInt(values.marksmanship, 10) || 0), weapon_bonus_limit = Math.min(weapon_bonus, weapon_bonus_max), weapon_skill_tot = attribute + skill, weapon_bonus_tot = weapon_skill_tot + weapon_bonus_limit + weapon_misc_attack, weapon_damage_tot = weapon_damage + weapon_misc_damage; setAttrs({ repeating_weapons_weapon_skill_tot : weapon_skill_tot, repeating_weapons_weapon_bonus_tot : weapon_bonus_tot, repeating_weapons_weapon_damage_tot : weapon_damage_tot }); console.log('>>>> weapon_skill_tot: '+weapon_skill_tot+' weapon_bonus_tot: '+weapon_bonus_tot+' weapon_damage_tot: '+weapon_damage_tot +' <<<<'); }); });
code that throws the log errors (there are a couple of other non-repeating attributes, but I've left them out for now to keep it simple.)
/* set weapon bonus and damage totals */ on("sheet:opened change:strength_total change:repeating_weapons", function () { console.log(">>>> Change Detected: Weapon Attacks - recalculating totals <<<<"); getAttrs(['repeating_weapons_weapon_skill', 'repeating_weapons_weapon_skill_tot', 'repeating_weapons_weapon_bonus', 'repeating_weapons_weapon_bonus_tot', 'repeating_weapons_weapon_misc_attack','repeating_weapons_weapon_bonus_max', 'repeating_weapons_weapon_damage', 'repeating_weapons_weapon_misc_damage', 'repeating_weapons_weapon_damage_tot', 'strength_total', 'agility_total', 'marksmanship', 'melee'], function (values) { const weapon_skill = parseInt(values.repeating_weapons_weapon_skill, 10) || 0, weapon_bonus = parseInt(values.repeating_weapons_weapon_bonus, 10) || 0, weapon_misc_attack = parseInt(values.repeating_weapons_weapon_misc_attack, 10) || 0, weapon_bonus_max = parseInt(values.repeating_weapons_weapon_bonus_max, 10) || 0, weapon_damage = parseInt(values.repeating_weapons_weapon_damage, 10) || 0, weapon_misc_damage = parseInt(values.repeating_weapons_weapon_misc_damage, 10) || 0, attribute = (weapon_skill === 0 ? parseInt(values.strength_total, 10) || 0 : parseInt(values.agility_total, 10) || 0), skill = (weapon_skill === 0 ? parseInt(values.melee, 10) || 0 : parseInt(values.marksmanship, 10) || 0), weapon_bonus_limit = Math.min(weapon_bonus, weapon_bonus_max), weapon_skill_tot = attribute + skill, weapon_bonus_tot = weapon_skill_tot + weapon_bonus_limit + weapon_misc_attack, weapon_damage_tot = weapon_damage + weapon_misc_damage; setAttrs({ repeating_weapons_weapon_skill_tot : weapon_skill_tot, repeating_weapons_weapon_bonus_tot : weapon_bonus_tot, repeating_weapons_weapon_damage_tot : weapon_damage_tot }); console.log('>>>> weapon_skill_tot: '+weapon_skill_tot+' weapon_bonus_tot: '+weapon_bonus_tot+' weapon_damage_tot: '+weapon_damage_tot +' <<<<'); }); });