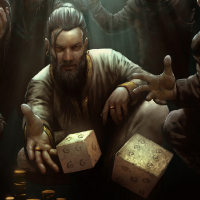
Here's my character sheet code (snippets): <span>Encumbrance:</span> <input type="number" value="0" name="attr_encumbrance"/>
<span>Base:</span> <input type="number" value="120" name="attr_movebase"/>
<span>Combat:</span> <input type="number" value="40" name="attr_movecombat"/>
<span>Charge/Run:</span> <input type="number" value="120" name="attr_moverun"/>
// When total ENCUMBRANCE changes, update the character's movement values...
on('change:encumbrance', function(eventInfo) {
getAttrs (['encumbrance', 'movebase', 'movecombat', 'moverun'], function(values) {
setAttrs(calcMovement(values.encumbrance));
});
});
// Helper function for calculating character's movement rates based on encumbrance
function calcMovement(theEncumbrance) {
// movement thresholds and values
const thresholds = [10, 7, 5, 0];
const moveBase = [30, 60, 90, 120];
const moveCombat = [10, 20, 30, 40];
const moveRun = [30, 60, 90, 120];
const index = thresholds.findIndex(element => theEncumbrance > element);
const returnValues = {};
returnValues['movebase'] = moveBase[index];
returnValues['movecombat'] = moveCombat[index];
returnValues['moverun'] = moveRun[index];
return returnValues;
}
// When a hidden ENCUMBRANCE field's value changes, update the character's overall encumbrance...
on('change:encarmor', function(eventInfo) {
getAttrs (['encumbrance', 'encarmor'], function(values) {
const newEncValue = values.encarmor;
setAttrs({
Encumbrance : newEncValue
});
});
}); I'm using the event handler and helper function to listen for a change in encumbrance and then set some movement values based on encumbrance thresholds. Everything works except for the case where the encumbrance value is "0". When this is the case, the helper fails - obviously(?) because a '0' encumbrance value returns '-1' from findIndex(). I've tried everything I can think of to set the index=0 when it returns '-1', but the form still breaks. And when I say break, depending on the approach, one or all of the movement fields stop responding and I have to completely refresh the sheet sandbox to get the character sheet functional again. I assume I'm dealing with a data type issue, but aren't familiar enough (yet) to be able to determine where and how to fix it. BTW, I've included the event handler that calculates the encumbrance value to begin with, at the bottom, in case the issues in there (when setting the sheet's attr_encumbrance value to '0').