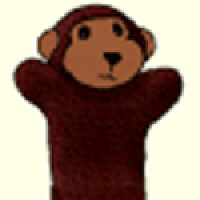
I found out today that the "silent" option on setAttrs is unreliable at best - if another call to setAttrs is made at roughly the same time, the first will not be silent. You can obviously code around this to some extent but it's possible to trigger the issue by tabbing between fields too quickly, if the change events associated with those fields call setAttrs (which I'm doing because I need to add bonuses to the base value entered by the player).
Here's an example sheet that illustrates the issue:
<div>Health (cascades): <input type="text" name="attr_health"></div><br><div>Mana: <input type="text" name="attr_mana"></div><br><div>Strength: <input type="text" name="attr_strength"></div><br>Entering a value in any field will add 1 to it, health will do this repeatedly, the other twofields won't cascade, unless the health field is already doing so. You can also enter a numberin the strength field, then enter a number in the mana field and hit tab twice quickly to seethe setAttrs for mana fire twice, even though "silent" is set to true on both calls<script type="text/worker">on("change:health", function(eventInfo) {setAttrs({ health: parseInt(eventInfo.newValue) + 1 });});on("change:mana", function(eventInfo) {setAttrs({ mana: parseInt(eventInfo.newValue) + 1 }, { silent: true });});on("change:strength", function(eventInfo) {setAttrs({ strength: parseInt(eventInfo.newValue) + 1 }, { silent: true });});</script>