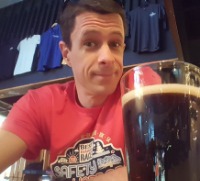
Hey there, fellow LDL holdouts! Some of you may have noticed that some of your maps with dynamic lighting are now producing "phantom" LoS blocking lines stretching to the upper left of your maps. This is related to a recent update that has broken LDL when "closed" polygons (startPt = endPt) are used. Since LDL is no longer supported and a fix is surely never coming, this little scriptlet converts your "closed" polygon into an "open" one which will not produce the phantom lines. For you folks knowledgeable with SVG paths, the script merely removes the last array element containing ["Z"] which denotes it as a closed polygon. To use, select the offending polygon path and run the following macro: !openpolygon Example gif (click to play). The rectangle on the DL layer is producing the phantom line prior to running the script but is functioning as expected afterward. Here's the code: const LDLOpenPolygon = (() => {
async function handleInput(msg) {
if (msg.type=="api" && msg.content.toLowerCase().indexOf("!openpolygon")==0) {
try {
let selected = msg.selected.filter(e => e._type==='path');
if (selected) {
let pathObj = getObj('path', selected[0]._id);
let pathArr = JSON.parse(pathObj.get("path"));
let closedPoly = pathArr.find(e => e[0] === 'Z') ? true : false;
if (closedPoly) {
let openPath = pathArr.filter(e => e[0] !== 'Z');
let pathObjCopy = JSON.parse(JSON.stringify(pathObj));
pathObjCopy._path = JSON.stringify(openPath);
let newPathObj = await createObj('path',pathObjCopy);
if (newPathObj) {
pathObj.remove();
} else {
sendChat('',`/w gm Unexpected error. The selected path was unaffected.`);
}
}
}
}
catch(err) {
sendChat('',`/w gm `+ 'Error: ' + err.message);
}
};
};
const registerEventHandlers = () => {
on('chat:message', handleInput);
};
on('ready', () => {
registerEventHandlers();
});
})();
If you are feeling frisky you could modify this to affect all paths on a given page or across your campaign, but there's no way I'm going to be held responsible for that if anything goes wrong! ;)