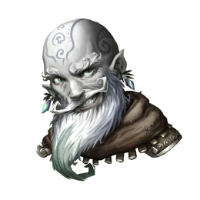
I'm trying to create a rolltemplate that displays the roll (a 1d100), the sum of the individual dice rolled (so if a roll is 23 the sum would be 5) and some other bits and bobs. Now, my issue is one of two things I think: I'm passing the calculated value (the 5 in the example above) incorrectly, or I'm making an error in how I display the value in the rolltemplate. I can't see which, or if it's something else. Please help! :) Rolltemplate: <rolltemplate class="sheet-rolltemplate-simple1d100template"> <div class="sheet-template-container"> <h2>{{name}}</h2> <div class="sheet-results"> <h3>Roll: {{roll}} </h3> <h3>Skill: {{skill}}</h3> <h3>Damage: {{damage}}</h3> <h3>Dmg: {{computed::damage}}</h3> </div> </div> </rolltemplate> Worker: //Roll an attack const std_roll = '1d100cs11cs22cs33cs44cs55cs66cs77cs88cs99'; const my_weapons = { weapon1: '&{template:simple1d100template} {{name=@{character_name} attacks with @{weapon1_name} }} {{roll=[[ '+std_roll+' ]]}} {{skill=@{weapon1_skill_value} }} {{damage=@{weapon1_damage} }} }', weapon2: '&{template:simple1d100template} {{name=@{character_name} attacks with @{weapon2_name} }} {{roll=[[ '+std_roll+' ]]}} {{skill=@{weapon2_skill_value} }} {{damage=@{weapon2_damage} }} }', weapon3: '&{template:simple1d100template} {{name=@{character_name} attacks with @{weapon3_name} }} {{roll=[[ '+std_roll+' ]]}} {{skill=@{weapon3_skill_value} }} {{damage=@{weapon3_damage} }} }', weapon4: '&{template:simple1d100template} {{name=@{character_name} attacks with @{weapon4_name} }} {{roll=[[ '+std_roll+' ]]}} {{skill=@{weapon4_skill_value} }} {{damage=@{weapon4_damage} }} }', }; const weapons = ['weapon1', 'weapon2', 'weapon3', 'weapon4']; const weapon_changes = weapons.map(weapon => `clicked:${weapon}`).join(' '); on(weapon_changes, eventInfo => { const trigger = eventInfo.triggerName.replace('clicked:',''); const roll_string = my_weapons[trigger]; console.log("Roll string "+roll_string); startRoll(roll_string, roll => { let result = String(roll.results.roll.result); const dmg = calculateDamage(result); console.log("Result "+result + " damage " +dmg); finishRoll( roll.rollId, { damage: dmg, } ); }); }); Calculator function: function calculateDamage(num) { let len = num.length; let sum = 0; switch(len){ case(1): //1-9 sum = parseInt(num); break; case(2): //10-99 let tens = parseInt(num.slice(0,1)); console.log("Tens "+tens); let ones = parseInt(num.slice(-1)); console.log("Ones "+ones); sum = tens+ones; console.log("Sum "+sum); break; case(3): //100 sum = 0; break; default: sum = 0; break; } console.log("Length "+len+" sum "+sum); return sum; }