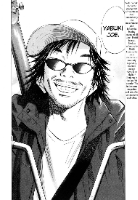
Since using parseInt is a popular input option, I wrote a simple function to parse input as rolls that might help other sheet developers. Given a string input, it parses it either as a number or a roll, depending on the content. It accepts all kinds of roll operations, and the roll needs to terminate in a number, a space, or a closed parenthesis; the result is returned, removing the spaces and uppercasing the dice
const parseRoll = ( roll ) => { const regex_int = / ^ (\s * [ \+\- ] ? \d {1,100} \s * ) $ / ; const regex_roll = / ^ ((\s * [ \+ \- \(\) ]\s * ) {0,4}? \d {0,10} [dD] ? \d {1,10} ((\s * [ \+ \- \*\/\(\) ]\s * ) {0,4}? \d {0,10} [dD] ? \d {1,10} ) * (\s * [ \) ]\s * ) {0,4}? ) + $ / if ( regex_int . test ( roll )) { return parseInt ( roll );} if ( regex_roll . test ( roll )) { return roll . replace ( /\s/ g , '' ). toUpperCase ();} return 0 ; } "Compute" the minimum and maximum of a roll, which is useful if you pass the value to a roll template for fumbles and critical. const maxRoll = (roll) => {
if ( isNaN ( Number ( roll )) == false ) { return roll ;} return parseRoll ( roll ). replace ( /[dD]/ g , '*' ) } const minRoll = ( roll ) => { if ( isNaN ( Number ( roll )) == false ) { return roll ;} return parseRoll ( roll ). replace ( /[dD]\d {1,100} / g , '' ) }