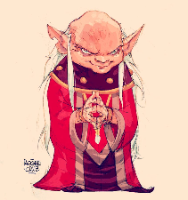
Hi, First up I'm not a programmer by background I'm just figuring things out as I go along so it's quite possible I'm doing something really dumb. I'm trying to make a quick script to sort all the cards drawn to make them easier to see. But I want to organise them by the deck they were drawn from and by name. To simplify things I've taken out the deck sorting and only sorting by name but still it's not doing what it should and the list is not sorted. I've tried different methods of sorting, originally using the .sort ( a, b ) method and most recently using underscore.js but it doesn't seem to make any difference no sorting actually happens. When I manually created an array of names and numbers and tried to sort that it worked as expected. So I'm not sure if my knowledge of JS is letting me down and I'm sorting an array that can't be sorted for some reason I don't know about. This is a snippet of my code. I'd appreciate if someone could tell me where I'm going wrong. if (msg.type === "api" && msg.content.indexOf("!sortCards") !== -1)
{
//store the page ID
const currentPage = Campaign().get('playerpageid');
//find all the cards on the page.
let cards = findObjs({
_type: 'graphic',
_subtype: 'card',
_pageid: currentPage,
layer: 'objects'
});
//Assign to each card graphic on the page the card name and deck name stored in name and bar1_max. Also resizes the card to make it smaller than default size.
for (i = 0; i< cards.length; i++)
{
let cardGraphic = cards[i];
let card = getObj('card', cardGraphic.get('_cardid'));
let deck = getObj('deck', card.get('_deckid'));
cardGraphic.set({
name: card.get('name'),
bar1_max: deck.get('name'),
height: 288,
width: 180
})
}
//Sort the cards by name and deck. NB deck sorting currently removed, only sorting by name. Trying to get something to work.
let sortedCards = _.sortBy(cards, 'name');
log(cards);
log(sortedCards);