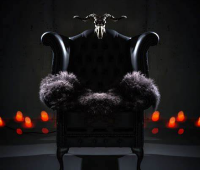
Keeping track of conditions has always been one of the more difficult things to take account for in D&D 4E and I have been seeking a way to accurately keep tabs in game, without having to bounce back and forth between a notepad file. I modified an old script by Aaron W. Token Status Reminder, and made it a little more cohesive to the broad spectrum of what is needed in D&D 4E.
on('ready', function(){ 'use strict'; on ("change:campaign:turnorder", function(obj) { var turn_order = JSON.parse(Campaign().get('turnorder')) || [], current = {}, currentMsg; current.turn=turn_order[0]; current.token = getObj( "graphic", current.turn.id); current.character = getObj('character', current.token.get("_represents")); current.markers = { marked : current.token.get("status_redmarker"), afflicted : current.token.get("status_bluemarker"), affliction : findObjs({_type:'attribute',_characterid:current.character.get('_id'),name:'affliction'})[0], ongoing : current.token.get("status_greenmarker"), ongoingDmg : findObjs({_type:'attribute',_characterid:current.character.get('_id'),name:'ongoing'})[0], bloodied : (current.token.get("bar1_value") < (Math.ceil(current.token.get("bar1_max") / 2))), slowed : current.token.get("status_snail"), dazed : current.token.get("status_spanner"), immobilized : current.token.get("status_padlock"), weakened : current.token.get("status_broken-shield"), blinded : current.token.get("status_bleeding-eye"), dominated : current.token.get("status_half-haze"), restrained : current.token.get("status_grab"), prone : current.token.get("status_back-pain"), stunned : current.token.get("status_fist"), unconscious : current.token.get("status_sleepy"), deafened : current.token.get("status_edge-crack"), defense : current.token.get("status_pink"), defensePen : findObjs({_type:'attribute',_characterid:current.character.get('_id'),name:'defense'})[0], toHit : current.token.get("status_yellow"), toHitPen : findObjs({_type:'attribute',_characterid:current.character.get('_id'),name:'toHit'})[0], endOnTurn : current.token.get("status_brown"), endOnTurnMg : findObjs({_type:'attribute',_characterid:current.character.get('_id'),name:'endOnTurn'})[0], grabbed : current.token.get("status_interdiction") }; current.name = current.token.get("name"); current.type = (current.character.get("controlledby") === "") ? 'Monster' : 'Player'; current.flagged = function(){ return (this.markers.marked || this.markers.afflicted || this.markers.ongoing || this.markers.bloodied ||this.markers.slowed || this.markers.dazed || this.markers.immobilized || this.markers.weakened || this.markers.blinded || this.markers.dominated || this.markers.restrained ||this.markers.prone ||this.markers.grabbed ||this.markers.unconscious ||this.markers.deafened || this.markers.defense || this.markers.toHit || this.markers.endOnTurn || this.markers.stunned); }; // End current turn sendChat('system','/e changes turn.'); //Begin Turn currentMsg = "Is up to bat."; if (current.flagged()){ currentMsg += "<ul>"; if(current.markers.marked){ currentMsg += "<li>" + current.type + " is <strong>Marked</strong>!</li>"; } if(current.markers.afflicted){ if(current.markers.affliction !== undefined){ currentMsg += "<li>" + current.type + " is <strong>afflicted with " + current.markers.affliction.get('current') + "</li>"; } else { currentMsg += "<li>" + current.type + " is <strong>afflicted</strong>!</li>"; } } if(current.markers.ongoing){ if(current.markers.ongoingDmg !== undefined){ currentMsg += "<li>" + current.type + " is taking <strong>ongoing damage by " + current.markers.ongoingDmg.get('current') + "</li>"; } else { currentMsg += "<li>" + current.type + " is taking <strong>ongoing damage</strong>! Take Damage?</li>"; } } if(current.markers.defense){ if(current.markers.defensePen !== undefined){ currentMsg += "<li>" + current.type + " is taking <strong>defense penalty with " + current.markers.defensePen.get('current') + "</li>"; } else { currentMsg += "<li>" + current.type + " is <strong>defense</strong>!</li>"; } } if(current.markers.toHit){ if(current.markers.toHitPen !== undefined){ currentMsg += "<li>" + current.type + " is taking <strong>a to hit penalty with " + current.markers.toHitPen.get('current') + "</li>"; } else { currentMsg += "<li>" + current.type + " is <strong>toHit</strong>!</li>"; } } if(current.markers.endOnTurn){ if(current.markers.endOnTurnMg !== undefined){ currentMsg += "<li>" + current.type + " is taking <strong>a penalty that ends on " + current.markers.endOnTurnMg.get('current') + "</li>"; } else { currentMsg += "<li>" + current.type + " is <strong>toHit</strong>!</li>"; } } if(current.markers.bloodied){ currentMsg += "<li>" + current.type + " is <strong>bloodied</strong>!</li>"; } if(current.markers.slowed){ currentMsg += "<li>" + current.type + " is <strong>slowed</strong>!</li>"; } if(current.markers.dazed){ currentMsg += "<li>" + current.type + " is <strong>dazed</strong>!</li>"; } if(current.markers.immobilized){ currentMsg += "<li>" + current.type + " is <strong>immobilized</strong>!</li>"; } if(current.markers.weakened){ currentMsg += "<li>" + current.type + " is <strong>weakened</strong>!</li>"; } if(current.markers.blinded){ currentMsg += "<li>" + current.type + " is <strong>blinded</strong>!</li>"; } if(current.markers.dominated){ currentMsg += "<li>" + current.type + " is <strong>dominated</strong>!</li>"; } if(current.markers.restrained){ currentMsg += "<li>" + current.type + " is <strong>restrained</strong>!</li>"; } if(current.markers.prone){ currentMsg += "<li>" + current.type + " is <strong>prone</strong>!</li>"; } if(current.markers.stunned){ currentMsg += "<li>" + current.type + " is <strong>stunned</strong>!</li>"; } if(current.markers.grabbed){ currentMsg += "<li>" + current.type + " is <strong>grabbed</strong>!</li>"; } if(current.markers.unconscious){ currentMsg += "<li>" + current.type + " is <strong>unconscious</strong>!</li>"; } if(current.markers.deafened){ currentMsg += "<li>" + current.type + " is <strong>deafened</strong>!</li>"; } currentMsg += "</ul>"; } sendChat(current.name, currentMsg); }); });
The following ChatSetAttr macros are used to manage the conditions that include messages;
!setattr --sel --affliction|?{Message|0}
!setattr --sel --ongoing|?{Message|0}
!setattr --sel --defensePen|?{Message|0}
!setattr --sel --toHit|?{Message|0}
!setattr --sel --endOnTurn|?{Message|0}
At the beginning of each turn, output looks like this;