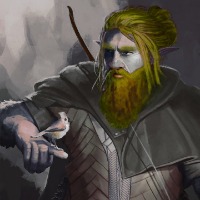
I've created what I thought was a simple script for my campaign that would let me keep track of the in-game time with a few commands.
The commands are !ttsethour, !ttsetmin and !ttsetsec, which set the hour, the minute of the hour, and the second of the minute. A fourth command, !time, will tell the three values separated by colons.
My problem is that the variables I set for the three values become undefined outside of their respective functions, and I'm having no luck trying to store the variables globally for use. I'm quite novice at Javascript. Any help would be greatly appreciated.
EDIT: To clarify, my output when I use !time is this: Time: undefined:undefined:undefined.
The code thus far:
https://gist.github.com/7ca2560fdbeecb8c049f.git
The commands are !ttsethour, !ttsetmin and !ttsetsec, which set the hour, the minute of the hour, and the second of the minute. A fourth command, !time, will tell the three values separated by colons.
My problem is that the variables I set for the three values become undefined outside of their respective functions, and I'm having no luck trying to store the variables globally for use. I'm quite novice at Javascript. Any help would be greatly appreciated.
EDIT: To clarify, my output when I use !time is this: Time: undefined:undefined:undefined.
The code thus far:
https://gist.github.com/7ca2560fdbeecb8c049f.git