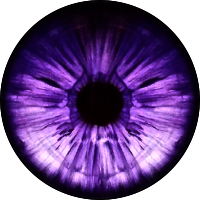
Token Status is a simple, no frills script that watches a single token bar for numerical changes, such as gaining or losing hit points from an attack or healing spell. It also includes a simple AoE blast token script you can see in use here . Basically just setting the size in units up to 9 with a status icon you can choose (default chemical-blast). TokenStatus monitors the bar you set as HealthBarID (1, 2, or 3) and will add the half-heart icon or set a red aura on the token once the token has less than half its maximum hit points. If a token drops below zero, it will add a Red X if the token doesn't represent a character sheet and does not have the isPC attribute set to true. To take advantage of the death save tracking... you must add the isPC attribute to your player's character sheets and set it to true . The tokens being used by the player's must also be representing those character sheets. When all this is set up properly, the script will add a skull icon and the angel-outfit icon to a pc's token when that token hits zero or less hit points. When the pc makes a death save, you can increment the number of fails or successes with !ds-pass or !ds-fail while selecting the token. Some Target and Pointer Tokens as a Bonus! Summary of Features Sets a dark red aura of the half-heart icon if a token drops below half its maximum hit points. Adds a Red X or death save tracking icons if the token drops to or below zero hit points. If negative hp tracking is turned off, the current hit points are maintained at zero. Prevents setting current hit points greater than the maximum hit points. Adds two commands for adding death save failures and successes to a selected token. !ds-pass adds one to the current death save successes (angel-outfit icon) !ds-fail adds one to the current death save failures (skull icon) Adds an option for quickly adjusting the aura of a token named BlastTarget. Size of the aura is set by hitting a number key while mousing over the chemical-blast icon on the token settings. Zero turns off the aura while one through nine sets the aura that many units big. // CONFIGURATION SETTINGS
var HealthBarID = 3;
var TrackNegativeHP = false;
var CanBeBloodied = true;
var UseAura = false;
var BloodiedIcon = "half-heart";
var BlastIcon = "chemical-bolt";
var SquareAura = false;
// API COMMAND HANDLER
on("chat:message", function(msg) {
if (msg.type !== "api") return;
if (msg.content.split(" ", 1)[0] === "!ds-pass") {
var Token = getObj("graphic", msg.selected[0]["_id"]);
if (Token.get("status_angel-outfit") !== false) {
if (Token.get("status_angel-outfit") === true) Token.set("status_angel-outfit", "1");
else if (Token.get("status_angel-outfit") === "1") Token.set("status_angel-outfit", "2");
else if (Token.get("status_angel-outfit") === "2") Token.set("status_angel-outfit", "3");
}
}
if (msg.content.split(" ", 1)[0] === "!ds-fail") {
var Token = getObj("graphic", msg.selected[0]["_id"]);
if (Token.get("status_skull") !== false) {
if (Token.get("status_skull") === true) Token.set("status_skull", "1");
else if (Token.get("status_skull") === "1") Token.set("status_skull", "2");
else if (Token.get("status_skull") === "2") {
Token.set("status_skull", "3");
Token.set("status_dead", true);
}
}
}
});
on("change:token", function(obj, prev) {
// Exit the script if the object is not a token or is a drawing...
if (obj.get("subtype") != "token" || obj.get("isdrawing") == "true") return;
// Check for the specified status icon on the BlastTarget and add an aura equal
// to the number set on the status.
if (obj.get("name") == "BlastTarget") {
var AuraRadius = parseInt(obj.get("status_" + BlastIcon));
var Scale = parseInt(getObj("page", obj.get("pageid")).get("scale_number"));
if (obj.get("statusmarkers").indexOf(BlastIcon + "@") != -1) {
obj.set("aura1_radius", AuraRadius * Scale);
obj.set("aura1_color", "#990000");
obj.set("aura1_square", SquareAura);
obj.set("showplayers_aura1", true);
}
if (AuraRadius == false || obj.get("statusmarkers").indexOf(BlastIcon + "@") == -1) {
obj.set("aura1_radius", "");
obj.set("status_" + BlastIcon, true);
}
} else {
// Get the tokens previous, current, and max hit points...
var PreviousHP = parseInt(prev["bar" + HealthBarID + "_value"]);
var CurrentHP = parseInt(obj.get("bar" + HealthBarID + "_value"));
var MaxHP = parseInt(obj.get("bar" + HealthBarID + "_max"));
// Exit if CurrentHP did not change...
if (PreviousHP === CurrentHP) return;
// Check for PC status...
if (obj.get("represents") != "") {
var isPC = getAttrByName(obj.get("represents"), "isPC");
}
// Get updated CurrentHP value to check for bloodied/dead status...
CurrentHP = obj.get("bar" + HealthBarID + "_value");
// If the token has more than zero hit points, remove the dying/death status icons...
if (CurrentHP > 0) {
obj.set("status_dead", false);
obj.set("status_skull", false);
obj.set("status_angel-outfit", false);
}
// If CanBeBloodied is true, check to see if the token is at half or less hp...
if (CanBeBloodied == true && CurrentHP <= MaxHP / 2) {
if (UseAura == true) {
obj.set("aura1_radius", "0");
obj.set("aura1_color", "#990000");
obj.set("aura1_square", SquareAura);
obj.set("showplayers_aura1", true);
} else {
obj.set("status_" + BloodiedIcon, true);
}
} else if (CanBeBloodied == true && CurrentHP > MaxHP / 2) {
if (UseAura == true) {
obj.set("aura1_radius", "");
} else {
obj.set("status_" + BloodiedIcon, false);
}
}
// Deal with hp less than or equal to zero...
if (CurrentHP <= 0 && MaxHP > 0) {
// If the token represents a PC, add skull and angel-outfit. Otherwise
// add the Red X...
if (isPC === undefined) {
obj.set("status_dead", true);
} else {
if (obj.get("status_skull") == false) obj.set("status_skull", true);
if (obj.get("status_angel-outfit") == false) obj.set("status_angel-outfit", true);
}
// Set CurrentHP to zero if negative hp tracking is off...
if (TrackNegativeHP == false) obj.set("bar" + HealthBarID + "_value", 0);
}
// Set current hp to maximum hp if current hp is greater than maximum hp...
if (CurrentHP > MaxHP) obj.set("bar" + HealthBarID + "_value", MaxHP);
}
});