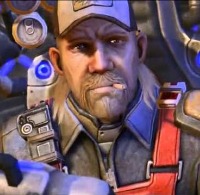
I'm trying to use a select drop down to change the active state of an array of other attributes, anyone happen to have an example something like this?
on("change:logos1_gettinggoodatthis_select", function(eventInfo) { if(eventInfo.newValue == "none") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "convince") setAttrs({ logos1_convince_dynamite: 1, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "changethegame") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 1, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "facedanger") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 1, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "gotoetotoe") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 1, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "hitwallyougot") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 1, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "investigate") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 1, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "sneakaround") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 1, logos1_taketherisk_dynamite: 0, }); if(eventInfo.newValue == "taketherisk") setAttrs({ logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 1, }); });
on("change:logos1_gettinggoodatthis_select", function(eventInfo) { let convince = 0, changethegame = 0, facedanger = 0, gotoetotoe = 0, hitallyougot = 0, investigate = 0, sneakaround = 0, taketherisk = 0; switch(eventInfo.newValue) { case "convince": convince = 1; break; case "changethegame": changethegame = 1; break; case "facedanger": facedanger = 1; break; case "gotoetotoe": gotoetotoe = 1; break; case "hitwallyougot": hitwallyougot = 1; break; case "investigate": investigate = 1; break; case "sneakaround": sneakaround = 1; break; case "taketherisk": taketherisk = 1; break; } setAttrs({ logos1_convince_dynamite: convince, logos1_changethegame_dynamite: changethegame, logos1_facedanger_dynamite: facedanger, logos1_gotoetotoe_dynamite: gotoetotoe, logos1_hitallyougot_dynamite: hitwallyougot, logos1_investigate_dynamite: investigate, logos1_sneakaround_dynamite: sneakaround, logos1_taketherisk_dynamite: taketherisk, }); });You dont need to use switch, you could use If as you did, but the key takeaway is since you are only changing one value in each if statement, you can establish variables to hold the values at the top, run through your ifs changing only the one value you need in each, and then use a single setAttrs statement at the end.
on("change:logos1_gettinggoodatthis_select", function() { getAttrs(['logos1_gettinggoodatthis_select'],function(values) { let convince = 0, changethegame = 0, facedanger = 0, gotoetotoe = 0, hitallyougot = 0, investigate = 0, sneakaround = 0, taketherisk = 0; let selected = values.logos1_gettinggoodatthis_select; if (selected === "convince") convince = 1; else if (selected === "changethegame") changethegame = 1; else if (selected === "facedanger") facedanger = 1; else if (selected === "gotoetotoe") gotoetotoe = 1; else if (selected === "hitwallyougot") hitwallyougot = 1; else if (selected === "investigate") investigate = 1; else if (selected === "sneakaround") sneakaround = 1; else if (selected === "taketherisk") taketherisk = 1; setAttrs({ logos1_convince_dynamite: convince, logos1_changethegame_dynamite: changethegame, logos1_facedanger_dynamite: facedanger, logos1_gotoetotoe_dynamite: gotoetotoe, logos1_hitallyougot_dynamite: hitwallyougot, logos1_investigate_dynamite: investigate, logos1_sneakaround_dynamite: sneakaround, logos1_taketherisk_dynamite: taketherisk, }); }); });And finally, the way you've named your attributes makes it very easy to use an even more elegant approach.
on("change:logos1_gettinggoodatthis_select", function() { getAttrs(['logos1_gettinggoodatthis_select'],function(values) { let selected = values.logos1_gettinggoodatthis_select; let settings = { logos1_convince_dynamite: 0, logos1_changethegame_dynamite: 0, logos1_facedanger_dynamite: 0, logos1_gotoetotoe_dynamite: 0, logos1_hitallyougot_dynamite: 0, logos1_investigate_dynamite: 0, logos1_sneakaround_dynamite: 0, logos1_taketherisk_dynamite: 0, }; let thisSetting = 'logos1_' + selected + '_dynamite'; if (settings[thisSetting] !== undefined) settings[thisSetting] = 1; setAttrs(settings); }); });
on("change:logos1_whostheboss_selectA change:logos1_whostheboss_selectB", function() { getAttrs(['logos1_whostheboss_selectA', 'logos1_whostheboss_selectB'],function(values) { let convince = 0, changethegame = 0, facedanger = 0, gotoetotoe = 0, hitallyougot = 0, investigate = 0, sneakaround = 0, taketherisk = 0; let selectedA = values.logos1_whostheboss_selectA; let selectedB = values.logos1_whostheboss_selectB; if (selectedA === "convince" || selectedB === "convince") convince = 1; else if (selectedA === "changethegame" || selectedB === "changethegame") changethegame = 1; else if (selectedA === "facedanger" || selectedB === "facedanger") facedanger = 1; else if (selectedA === "gotoetotoe" || selectedB === "gotoetotoe") gotoetotoe = 1; else if (selectedA === "hitwallyougot" || selectedB === "hitwallyougot") hitwallyougot = 1; else if (selectedA === "investigate" || selectedB === "investigate") investigate = 1; else if (selectedA === "sneakaround" || selectedB === "sneakaround") sneakaround = 1; else if (selectedA === "taketherisk" || selectedB === "taketherisk") taketherisk = 1; setAttrs({ "logos1_whostheboss_convince_dynamite": convince, "logos1_whostheboss_changethegame_dynamite": changethegame, "logos1_whostheboss_facedanger_dynamite": facedanger, "logos1_whostheboss_gotoetotoe_dynamite": gotoetotoe, "logos1_whostheboss_hitallyougot_dynamite": hitwallyougot, "logos1_whostheboss_investigate_dynamite": investigate, "logos1_whostheboss_sneakaround_dynamite": sneakaround, "logos1_whostheboss_taketherisk_dynamite": taketherisk, }); }); });
if (selectedA === "convince" || selectedB === "convince") convince = 1; if (selectedA === "changethegame" || selectedB === "changethegame") changethegame = 1; if (selectedA === "facedanger" || selectedB === "facedanger") facedanger = 1; if (selectedA === "gotoetotoe" || selectedB === "gotoetotoe") gotoetotoe = 1; if (selectedA === "hitwallyougot" || selectedB === "hitwallyougot") hitwallyougot = 1; if (selectedA === "investigate" || selectedB === "investigate") investigate = 1; if (selectedA === "sneakaround" || selectedB === "sneakaround") sneakaround = 1; if (selectedA === "taketherisk" || selectedB === "taketherisk") taketherisk = 1;