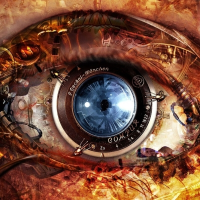
Are you sick and tired of making sheet workers? Of copying the same sheet worker over and over, changing just a few things in the first and last lines? Would you like to add any number of sheet workers with just a single line of easy to read code for each? Have I got the tool for you! Call now for your special offer, terms & conditions may apply, don't read the small print. Seriously though, I am really excited with my newest tool. I already created the wiki page Universal Sheet Workers, to explain some ways to streamline making repetitive sheet workers, but that can be pretty clunky and impenetrable to people not used to heavy coding. But this tool - this is (I think) simpler to use, and more powerful, so takes that to a whole new dimension. Hence the silly name Multiversal Sheet Workers! This is a script that you drop into any character sheet's script block, of around 100 lines of code. Then once in, it generates sheet workers for you. You simply fill two variables: multistats, and multifunctions, with some information (described later), and it builds the sheet workers for you. In my test sheet, I replaced 120 sheet workers, totalling around 800 lines of code, with about 50 lines of instructions in total. Of course, I was able to use loops, arrow functions, and various other tricks to handle multiple sheet workers with a single line, but even at 1-2 lines per sheet worker, it would be a huge saving of work. I've reserved the following posts to explain how to use it. Once I've refined it through testing, and people hopefully using it, I'll add it to the wiki. A quick summary: add all the code from post into your sheet's script block add attribute lists to the multistats variable. add functions to the multifunction variable. and you're done. Post 2 is the Multiversal code. Post 3 is a reference post, describing briefly how to set up the two special objects (multistats and multifunctions). And also things to avoid, when you shouldnt use multiversals, etc. Post 4 explains the multistats object, again with detailed examples. Post 5 explains the multifunction object in detail, with examples. this is likely to be the scariest part of setup, but it's much simpler than it appears. This is also the post that includes a good explanation of what is happening under the hood. So if you read anything, the first half of the post is a good start. Post 6: a hypothetical solution of how you would convert an entire character sheet to sheet workers, using advanced tips to make the code really short. It describes how to name your attributes to get the best out of this. Post 7 is reserved for notes on version updates, or any extra details i think off that dont fit in the previous posts. I'm really hoping people find this as useful as I do :) PS: jump to Wes's post for a great example of how to use this script, showing how simple it is.