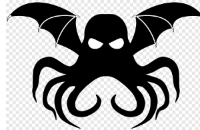
I wish I could have thanked Cuerpo but the thread is auto closed. This code works fantastic!
Community Forums: [Script] Bloodied and Dead Status Markers - Extended | Roll20: Online virtual tabletop
https://app.roll20.net/forum/permalink/725584/
I wanted to extend a small change, that works with my preferred setup (bar 3 is health, bar 2 is ac, bar 1 is npc cr), but also work with existing monsters added by module.
// Auto select bar1 or bar3 // Is life bar set at all? // If not simply escape the function. if(obj.get("bar1_max") === "") { if(obj.get("bar3_max") === "") { return; } else { lifeBarNumber = 3; } } else { lifeBarNumber = 1; }
The full script would then
on("change:graphic", function(obj) { // Settings: //var lifeBarNumber = 3; // 3:red; 2:blue; 1:green var lifeBarNumber = 0; // 3:red; 2:blue; 1:green var percentForRedmark = 50; // Write for example 50 for 50% var totalKillThreshold = -10; // As soon as a tokens life goes bellow this value // it gets an additional (killed) symbol. // Auto select bar1 or bar3 // Is life bar set at all? // If not simply escape the function. if(obj.get("bar1_max") === "") { if(obj.get("bar3_max") === "") { return; } else { lifeBarNumber = 3; } } else { lifeBarNumber = 1; } // Back from the dead? if((obj.get("status_dead") == true || obj.status_skull == true) && obj.get("bar" + lifeBarNumber + "_value") > 0){ SetStatuSymbolById(obj,3,1); } // Total-kill if(obj.get("bar" + lifeBarNumber + "_value") <= totalKillThreshold){ SetStatuSymbolById(obj,0,0); SetStatuSymbolById(obj,1,1); SetStatuSymbolById(obj,2,1); SetStatuSymbolById(obj,3,0); } // Dead else if(obj.get("bar" + lifeBarNumber + "_value") <= 0){ SetStatuSymbolById(obj,0,0); SetStatuSymbolById(obj,1,1); SetStatuSymbolById(obj,2,0); SetStatuSymbolById(obj,3,0); } // Bellow x% life else if(obj.get("bar" + lifeBarNumber + "_value") <= obj.get("bar" + lifeBarNumber + "_max") / 100 * percentForRedmark) { SetStatuSymbolById(obj,0,1); SetStatuSymbolById(obj,1,0); SetStatuSymbolById(obj,2,0); } else{ ResetDeathSymbols(obj); // Back from the dead but now again with full (or beyond that) health? // TODO: >= not working..? if(obj.get("bar" + lifeBarNumber + "_value") == obj.get("bar" + lifeBarNumber + "_max")){ SetStatuSymbolById(obj,3,0); } } }); /* Reset the following symboles redmarker, dead & skull */ function ResetDeathSymbols(obj){ SetStatuSymbolById(obj,0,0); SetStatuSymbolById(obj,1,0); SetStatuSymbolById(obj,2,0); } /* Sets the value of a given tokens symbol by the symbols id symbolid: 0:redmarker 1:dead 2:skull 3:death-zone x:y value: true/1 or false/0 */ function SetStatuSymbolById(obj, symbolid, value) { switch(symbolid){ case 0: SetStatusSymbolByName(obj, "redmarker", value); break; case 1: SetStatusSymbolByName(obj, "dead", value); break; case 2: SetStatusSymbolByName(obj, "skull", value); break; case 3: SetStatusSymbolByName(obj, "death-zone", value); break; default: // Something is wrong so log it and exit. log("Error: SetStatuSymbolById - There was no usable number for symbolid given!"); log(" Object name: " + obj.get("name") + "; symbolid: " + symbolid + "; value: " + value); return; } } /* Sets the value of a given tokens symbol by the symbols name value: true/1 or false/0 */ function SetStatusSymbolByName(obj, symbolname, value){ value = CheckForTrueOrFalse(value); // Set the symbols value. obj.set("status_" + symbolname, value); } /* Checks if a given value is true or false. value: 0:false 1:true default:false */ function CheckForTrueOrFalse(value){ if (value === 0){value = false;} else if (value === 1){value = true;} else if (value === true || value === false){ // Nothing to do, these are valid values. } // The input for value is not known, therefore we set it to false. else{ value = false; log("Error: CheckForTrueOrFalse - An unhandled value has been used!"); log(" value has been set to de default value " + value); } return value; } /* Debug function Place where ever you would like to see the actual values. */ function DebugMe(obj,msg){ log("--- DEBUG: " + msg + "---"); log("obj name: " + obj.get("name")); log("status values:"); log("redmarker: " + obj.get("status_redmarker")); log("dead: " + obj.get("status_dead")); log("skull: " + obj.get("status_skull")); log("death: " + obj.get("status_death")); }