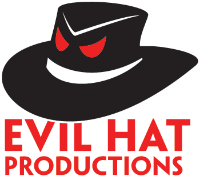
On the new sheet I'm working on, players are able to choose a skill set that when chosen automatically adds a bunch of skills to their skill list. The skill list is a repeating field set. I've noticed that every now and then — it's not consistent and not easily reproducible — one or more skills in a given set are omitted when this is done. I figured I'd done something boneheaded in my code, but never managed to find out why, until today. The first comment I'd expect to get is that I must be running into some sort of asynchronous problem, but generateRowID() is a synchronous function, and no async functions are involved within the for loop that generates the IDs: var newset = [ "Skill", "Skill", "Skill", "Skill", "Skill", "Skill", "Skill", "You get the idea" ];
var attrs = {};
for(var i=0; i < newset.length; i++) {
var newid = generateRowID();
var skillid = "repeating_skills_" + newid + "_skill";
attrs[skillid] = skillname;
attrs["repeating_skills_" + newid + "_rating"] = 0;
}
setAttrs(attrs);
So to chase this down I put a console logger in there that showed me the skillid value for each skill it was inserting. I went along for a while, then noticed the bug had happened again, leaving out the Networking skill when I loaded a particular list. Here's what the logger told me: VM4:361 INSERTED: repeating_skills_-MAqQ4bz1ezsMkjSAigZ_skill Networking
VM4:361 INSERTED: repeating_skills_-MAqQ4bz1ezsMkjSAigZ_skill Operate
Yup; those IDs are identical, indicating that the same value was returned by two successive calls to generateRowID(). The entry from Operate overwrote the entry for Networking because they both got the same ID number for their row. For the time being, I'm using this workaround: var uniqueids = {};
var generateActuallyUniqueRowID = function() {
var generated = generateRowID();
while ( uniqueids[generated] === true ) {
console.log("generateActuallyUniqueRowID: " + generated + " is not a unique ID, trying again.");
generated = generateRowID();
}
uniqueids[generated] = true;
return generated;
}
But really, shouldn't generateRowID() behave in such a way that it's not needed?