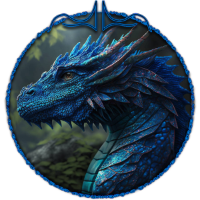
I have a api script that outputs a random result of drinking a potion, but it outputs the result to everyone. I was hoping to be able to just output it to the character I select. The current output looks like this: var PEL1 = (function()
{
'use strict';
const positiveEffectLevel1 =
[
{low: 1, high: 1, result: "Sense other Fey within 2 miles."},
{low: 2, high: 2, result: "Find Water within 2 miles."},
{low: 3, high: 3, result: "Detect poisons."},
{low: 4, high: 4, result: "Identify plants."},
{low: 5, high: 5, result: "Sense weather."},
{low: 6, high: 6, result: "Cantrips: Roll on Cantrip Chart."}
];
function registerEventHandlers()
{
on('chat:message', PEL1.handleChatMessage);
}
/**
* Grab chat message objects
*
* @param {object} msg
*/
function handleChatMessage(msg)
{
// Check if we are dealing with a !critical command.
//if (msg.type === "api" && msg.content.indexOf("!critical") !== -1) //JDP
if (msg.type === "api" && msg.content.indexOf("!positiveEffectLevel1") !== -1)
{
var content = msg.content;
var words = content.split(' ');
// Sanity check
if (words.length > 0)
{
// Sanity check
if (words[0] === '!positiveEffectLevel1')
{
var rolled = 0;
// Was a roll amount given? If so parse the second "word" as an int, otherwise create a randomInteger.
if (words.length === 2)
{
rolled = parseInt(words[1]);
}
else
{
rolled = randomInteger(6);
}
// Sanity check
if (typeof rolled !== 'number' || rolled === 0)
{
rolled = randomInteger(6);
}
// Get the smack object as a smash variable
var smash = PEL1._determineCritical(rolled);
// Sanity check
if (smash)
{
// Send the critical result as a formatted string in chat
//sendChat('Critical Hit', rolled.toString() + "% <b>" + smash.result + "</b><br><i><b>Slash: </b>" + smash.slash + '</i><br><i><b>Blunt: </b>' + smash.blunt + '</i><br><i><b>Pierce: </b>' + smash.pierce + '</i>'); //JDP'
sendChat('Positive Effect Level 1', smash.result);
}
else
{
sendChat('Positive Effect Level 1', 'Invalid % roll given, or something went wrong. GM makes something up.');
}
}
}
}
}
/**
* Internal function given the roll value returns the object indicating the result and effect.
*
* @param {int} roll
* @return {object} smack
* @private
*/
function _determineCritical(roll)
{
// Use _.find to figure out what happened.
return _.find(positiveEffectLevel1, function (hit)
{
return (roll >= hit.low && roll <= hit.high);
});
}
return {
registerEventHandlers: registerEventHandlers,
handleChatMessage: handleChatMessage,
_determineCritical: _determineCritical
}
}()); But I would like to incorporate something like this so I can select the character I want to see it: /w ?{Whisper|Jethro Hammerstroke|Skylar Battlebend|Dahk Turnskull|Macy Maples|Flick|Arno|Aiden}