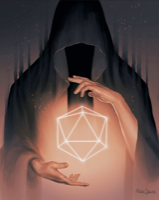
i am triing to modify a script and have it roll a roll automatically. sorry idk how to post in a more legible way. the bold part is the line in question and the underlined section of that part is the roll i am attempting to achieve. /* jshint undef: true */ /* globals sendChat, randomInteger, _, on */ var MFumble = (function() { 'use strict'; const Mfumble = [ {low: 1, high: 50, result: "Brilliant Recovery", effect: "No Fumble, Lucky You."}, {low: 51, high: 98, result: "Brilliant Recovery", effect: "No Fumble, Lucky You."}, {low: 99, high: 100, result: "Wild Surge", effect: "your magic goes awry consult wild surge table 1d10000 ."}, ]; function registerEventHandlers() { on('chat:message', MFumble.handleChatMessage); } /** * Grab chat message objects * * @param {object} msg */ function handleChatMessage(msg) { // Check if we are dealing with a !magicfumble command. if (msg.type === "api" && msg.content.indexOf("!Mfumble") !== -1) { var content = msg.content; var words = content.split(' '); // Sanity check if (words.length > 0) { // Sanity check if (words[0] === '!Mfumble') { var rolled = 0; // Was a roll amount given? If so parse the second "word" as an int, otherwise create a randomInteger. if (words.length === 2) { rolled = parseInt(words[1]); } else { rolled = randomInteger(100); } // Sanity check if (typeof rolled !== 'number' || rolled === 0) { rolled = randomInteger(100); } // Get the smack object as a smash variable var smash = MFumble._determineMFumble(rolled); // Sanity check if (smash) { // Send the critical result as a formatted string in chat sendChat('Magic Fumble', rolled.toString() + "% <b>" + smash.result + "</b><br><i><b>Effect: </b>" + smash.effect +"</b><br><i><b>Other: </b>" + smash.other + '</i>'); } else { sendChat('MFumble', 'Invalid % roll given, or something went wrong. GM makes something up.'); } } } } } /** * Internal function given the roll value returns the object indicating the result and effect. * * @param {int} roll * @return {object} smack * @private */ function _determineMFumble(roll) { // Use _.find to figure out what happened. return _.find(Mfumble, function (hit) { return (roll >= hit.low && roll <= hit.high); }); } return { registerEventHandlers: registerEventHandlers, handleChatMessage: handleChatMessage, _determineMFumble: _determineMFumble } }()); /** * Fires when the page has loaded. */ on("ready", function() { MFumble.registerEventHandlers(); });