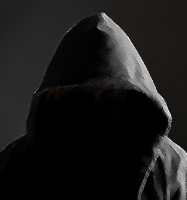
Hey, guys! So I have this issue. Roll20 character sheets don't actually store each attribute set in HTML until the attribute is actually changed from default value. This results in an error of " Cannot read property 'get' of undefined " when an API script uses obj.get() to get an attribute that hasn't yet been stored. I could make a sheet worker that actually sets each attribute in place at the default value (sort of circumventing the problem), but this seems like a bit of a kludge fix. Is there a way to have an obj.get() "fail gracefully" by, for example, somehow setting a fall-back value when you call for it ? (such as you can do with a sheetworker with "values.attribute || 0;" wherein the zero at the end after the "||" makes sure that a failed value resolution will result in a value of "0"). Thank you in advance for any help you can provide. Below is a snippet of code by way of example. This generated the error message above in the console when it ran, because the attribute "EqSlot" was not yet stored. It only existed as a default input value in the HTML of the page (e.g., name="attr_EqSlot" value="1"). But when I ran it after setting the attribute into the character sheet manually (under Attributes & Abilities), it worked fine. // Set ID
var sID = obj.get("_characterid");
// Get ability to be adjusted
var oSlot = findObjs({
type: 'attribute',
characterid: sID,
name: "EqSlot"
}, {caseInsensitive: false})[0];
var sSlot = oSlot.get("current");
var nSlot = parseInt(sSlot);